Below are the error messages from STS and Postman. Utilizing pertinent classes with their corresponding imports, the POST request was formulated. A potential source of the problem could be attributed to constructors, as the user harbors suspicion about potential oversight in annotations. While attempting to transmit a JSON request via REST API using Postman, the user stumbled upon an error, perplexed as to the origin of the mishap.
Unraveling Deserialization: A Guide to String-Argument Constructors
When working with JSON parsing, programmers often come across an error associated with the ObjectMapper class in the com.fasterxml.jackson.databind package. They may bump into a specific JsonMappingException that states “cannot construct instance of a certain class: no string-argument constructor/factory method to deserialize from string value (‘’)”.
Typically, such an error can emerge in a web application constructed with Spring MVC having an AngularJS front end, but it can also pop up in an all-Java program. This guide will take you through some examples to further solidify your understanding.
A Dive into Beans
Consider a standard application that utilizes beans. Two such beans are ‘Shipment’ and ‘Activity’, described below:
Shipment.java
Annotated with @JsonIgnoreProperties(ignoreUnknown = true), the ‘Shipment’ class has an ArrayList instance variable called activity. This variable stores the activity associated with the shipment. Here’s how it is structured:
@JsonIgnoreProperties(ignoreUnknown = true)
public class Shipment {
@JsonProperty("Activity")
private ArrayList activity;
public ArrayList getActivity() {
return activity;
}
public void setActivity(ArrayList activity) {
this.activity = activity;
}
}
Activity.java
Similarly, the ‘Activity’ class, also annotated with @JsonIgnoreProperties(ignoreUnknown = true), has an ArrayList instance activityLocation that stores the activity’s location. Its structure is as follows:
@JsonIgnoreProperties(ignoreUnknown = true)
public class Activity {
@JsonProperty("ActivityLocation")
private ArrayList activityLocation;
public ArrayList getActivityLocation() {
return activityLocation;
}
public void setActivityLocation(ArrayList activityLocation) {
this.activityLocation = activityLocation;
}
}
Understanding the above classes can help programmers navigate through the deserialization process more competently while decoding the error message. The key is to identify the type of error message and the related class, enabling faster troubleshooting and problem-solving.
Decoding ActivityLocation and Address Classes
To better understand and navigate the ‘no string-argument constructor/factory method’ error, it’s crucial to familiarize yourself with other beans utilized within the application. Here, we will delve into two more classes: ActivityLocation and Address.
ActivityLocation.java
The ActivityLocation class, like its counterparts Shipment and Activity, is annotated with @JsonIgnoreProperties(ignoreUnknown = true). This annotation helps in handling unrecognized or new fields in the JSON payload without throwing exceptions, thus enhancing our code’s compatibility with evolving JSON structures.
This class specifically deals with storing the Address of an activity. As such, it has a single instance variable address:
@JsonIgnoreProperties(ignoreUnknown = true)
public class ActivityLocation {
@JsonProperty("Address")
private Address address;
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
}
Through its getter and setter methods, the ActivityLocation class fetches and sets the Address respectively.
Address.java
The Address class, also marked with @JsonIgnoreProperties(ignoreUnknown = true), holds specific location details. It has three instance variables, namely city, stateProvinceCode, and countryCode.
@JsonIgnoreProperties(ignoreUnknown = true)
public class Address {
@JsonProperty("City")
private String city;
@JsonProperty("StateProvinceCode")
private String stateProvinceCode;
@JsonProperty("CountryCode")
private String countryCode;
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getCountryCode() {
return countryCode;
}
public void setCountryCode(String countryCode) {
this.countryCode = countryCode;
}
public String getStateProvinceCode() {
return stateProvinceCode;
}
public void setStateProvinceCode(String stateProvinceCode) {
this.stateProvinceCode = stateProvinceCode;
}
}
The Address class allows the extraction of detailed information about the location, including the city, state/province, and country, making it an essential part of the whole deserialization process.
It’s worth noting that understanding the structure and function of these classes can help coders to effectively track down and resolve the JsonMappingException error, promoting the smooth operation of their applications.
Navigating Through JSON Parsing
Taking a look at the accurate mapping of the JSON content through the provided code snippet will give us a comprehensive understanding of the deserialization process. It will also help us identify potential pain points that may lead to the “no string-argument constructor/factory method” error.
public class JsonMapping {
public static void main(String[] args) {
String jsonMessage = "" +
"{" +
" \"Activity\": [{" +
" \"ActivityLocation\": { " +
" \"Address\": { " +
" \"City\": \"Hana\", " +
" \"StateProvinceCode\": \"Hi\", " +
" \"CountryCode\": \"US\" " +
" } " +
" } " +
" }, " +
" { " +
" \"ActivityLocation\": { " +
" \"Address\": { " +
" \"City\": \"Honolulu\", " +
" \"StateProvinceCode\": \"Hi\", " +
" \"CountryCode\": \"US\" " +
" } " +
" } " +
" }]" +
"} ";
try {
ObjectMapper mapper = new ObjectMapper();
mapper.enable(DeserializationFeature.ACCEPT_SINGLE_VALUE_AS_ARRAY);
Shipment shipment = mapper.readValue(jsonMessage, Shipment.class);
System.out.println("shipment representation = " + shipment.toString());
} catch (Exception e) {
e.printStackTrace();
}
}
}
The challenge arises when there’s an attempt to modify the data inside the jsonMessage variable. More specifically, the issue lies in changing the JSON format from:
{
"ActivityLocation": {
"Address": {
"City": "Honolulu",
"StateProvinceCode": "Hi",
"CountryCode": "US"
}
}
To:
{
"ActivityLocation": {
"Address": ""
}
}
The error surfaces when an empty string replaces the Address object. It’s essential to note that the incoming data is controlled by a third party and can’t be modified.
Dissecting the Error
The error stems from the incompatibility between the received JSON format and the expected class structure. To elucidate, the Address class expects a populated Address node added in the ActivityLocation class. However, the incoming JSON only has an empty string under the Address field.
Overcoming the Challenge
Unfortunately, there’s no specific annotation that handles this particular scenario. Maintaining the expected JSON structure is crucial here. Should the Address field come as a string instead of a JSON object, it would naturally conflict with the class setup. This discrepancy between the Address class structure and the received JSON format is a common cause of the JsonMappingException.
There are several workarounds possible:
- Change the Address field type: If the incoming data format can’t be changed (as it’s controlled by a third party), consider modifying the Address field to a String type in the case when it’s empty;
- Create a custom deserializer: Another alternative is to build a specialized deserializer that can handle various formats. This deserializer will ascertain that even when the Address field comes empty, it is mapped to an empty Address object.
Being vigilant and understanding the incoming JSON structure plays a pivotal role in handling such exceptions. Always remember to align your class structures with the data format you expect to receive for a seamless deserialization process.
Unraveling Solutions to the Deserialization Error
Understanding the source of the “no string-argument constructor/factory method” error is crucial to resolving it. Here are some potential solutions:
Provision 1: Correct Method Call
Ensure to use the correct method readValue(), which is designed for JSON deserialization. Misuse, such as using convertValue() instead of readValue(), can lead to the error. Auto-completion by IDEs may lead to an inadvertent incorrect method call.
Provision 2: Configuration Tweaks
Configure the ObjectMapper to accept an empty string as a null object. This can be done by invoking DeserializationFeature.ACCEPT_EMPTY_STRING_AS_NULL_OBJECT.
For older Jackson versions:
mapper.configure(DeserializationConfig.Feature.ACCEPT_EMPTY_STRING_AS_NULL_OBJECT, true)
For newer versions:
mapper.enable(DeserializationFeature.ACCEPT_EMPTY_STRING_AS_NULL_OBJECT);
Provision 3: Substituting Empty String with Object Description
The error indicates the deserializer’s failure in locating a string-argument constructor for the Address class while deserializing from an empty string. To resolve this, replace the empty string with an object description enclosed in curly braces {}.
Provision 4: Ensuring Accurate JSON Formatting
Inaccurate JSON formatting, such as quotes wrapping an object definition, can also cause this exception.
Incorrect format:
{ "foo": "{ \"value\": 42}" }
Correct format:
{ "foo": { "value": 42 } }
It’s important to remember that identifying and understanding the root cause of the issue, and choosing the correct solution accordingly, plays a significant role in addressing the no string-argument constructor/factory method error. This leads to better programming practices and a smoother deserialization process.
Troubleshooting String Deserialization: Understanding the No String-Argument Constructor/Factory Method Error
When dealing with REST APIs and sending JSON requests using tools like Postman, it’s not uncommon to encounter errors. One such notable error is the no String-argument Constructor/Factory method when attempting to deserialize from String value (http://localhost:8080/categories/1). This error typically points to an issue with object deserialization.
Understanding the Error This error originates from the Jackson library in java, which is a powerful tool for binding JSON data to java objects. When the library attempts to translate a JSON object into a java object (deserialization), it requires the java object to have a constructor that matches the JSON. The no String-argument Constructor/Factory method error suggests that Jackson has failed to find a suitable constructor for the task.
The Root Cause The root cause of this exception is often a missing or misconfigured constructor in the Java class intended for deserialization. In this case, the Film class may not have the appropriate constructor which is causing Jackson to fail in deserializing and subsequently resulting in the error.
Examining the JSON Request A typical JSON request for creating a new Film resource with Postman using the POST method at the given URL (http://localhost:8080/films) would look like this:
{
"titre": "my titre8",
"description": "my descr8",
"realisateur": "realisateur8",
"categorie":"http://localhost:8080/categories/1"
}
Here, the categorie field’s value is a URL string.
Looking at the Film Class
The Film class is annotated with @Entity, implying that it’s a JPA entity. The class uses the Lombok library annotations like @Data, @AllArgsConstructor, @NoArgsConstructor, and @ToString to automatically generate boilerplate code.
@Entity
@Data @AllArgsConstructor @NoArgsConstructor @ToString
public class Film {
@Id @GeneratedValue(strategy= GenerationType.IDENTITY)
private Long id;
private String titre;
private String description;
private String realisateur;
private Date dateSortie;
private double duree;
private String photo;
@OneToMany(mappedBy="film")
private Collection projections;
@ManyToOne
private Categorie categorie;
}
Here, the Categorie attribute is a URL string, which could be the source of the error.
Keep in mind that while annotations like @AllArgsConstructor and @NoArgsConstructor simplify code, they may not always cater to all use-cases. You may need to manually define constructors or adjust your deserialization process to suit your specific needs.
Unveiling the Categorie Class
The Categorie class, part of the org.sid.cinema.entities package, is another entity class used in the project. It represents the ‘Category’ of the film and it’s designed as follows:
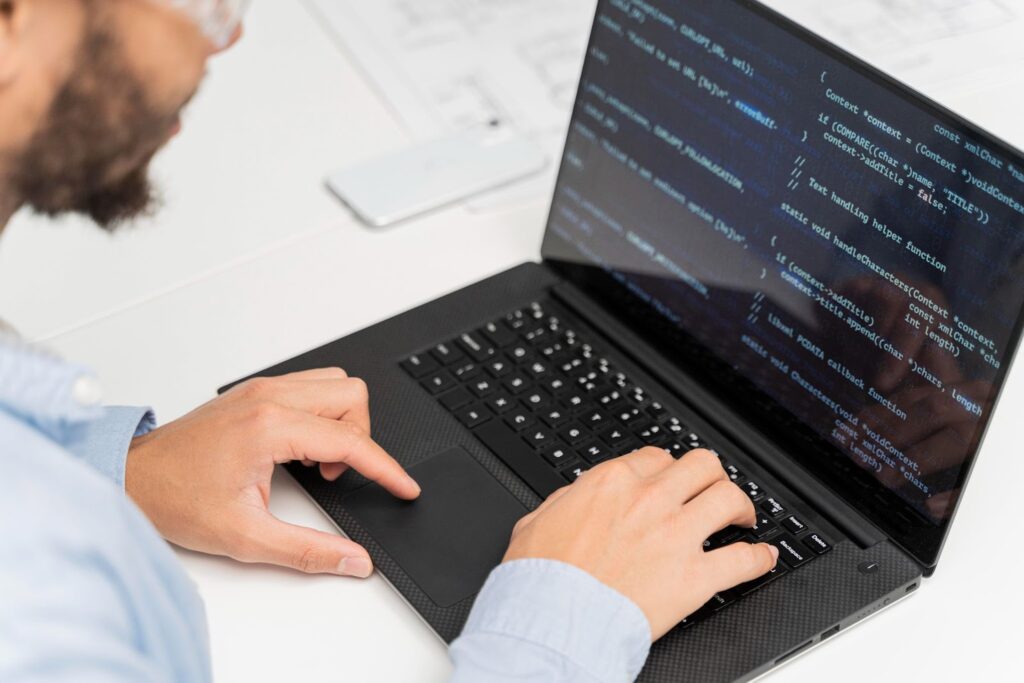
package org.sid.cinema.entities;
import java.util.Collection;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.OneToMany;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.ToString;
@Entity
@Data @AllArgsConstructor @NoArgsConstructor @ToString
public class Categorie {
@Id @GeneratedValue(strategy=GenerationType.IDENTITY)
private Long id;
@Column(length=75)
private String name;
@OneToMany(mappedBy="categorie")
private Collection films;
}
This class, similar to the Film class, is annotated with the @Entity and Lombok annotations. The Categorie class has a name attribute, which is only allowed to be 75 characters long according to @Column(length=75) annotation, and a films attribute that represents a collection of films that belong to a specific category.
Troubleshooting the Deserialization Error
The key problem causing the deserialization error is a type mismatch. The JSON request sent to the API is expected to have an Object of type Categorie for the categorie field. Instead, a string value is being provided, leading to the error.
The JSON request should be modified as follows for the correct data type:
{
"titre": "my titre8",
"description": "my descr8",
"realisateur": "realisateur8",
"categorie": {
"id": 1,
"name": "XX"
}
}
As you can see, the categorie field now consists of an object with id and name fields, rather than just a URL string. This aligns with the data type expected by the Film class and should resolve the deserialization error.
Dealing with LocalDate Deserialization
While quite similar to past problems, this issue is unique—it’s about transforming a string into a LocalDate object. The error is emanated from your Spring Tool Suite (STS), and it looks somewhat like this:
“Warning ID 6216 triggered at 12:47:04.507 on the 14th of December, 2018, during a code execution at port 8080. Warning indicates that the DefaultHandlerExceptionResolver managed to pinpoint an issue with deserialization—specifically the deserialization of the check-in date for a reservation request.”
The particular error that triggered this warning is the com.fasterxml.jackson.databind.JsonMappingException – suggesting that the creation of an instance of the ReservationRequest class has been unsuccessful. This was due to the absence of a constructor or factory method that can decode a string value (‘2018-12-14’).
According to the error log, this problem was found at the source input’s line 3, column 16. The input source was identified as a PushbackInputStream with an ID of 73ff9989.
In your case, Postman lays this problem out in the open with the following message:
“A JSON parse error has occurred due to the inability to deserialize a string (‘2018-12-14’) into a java.time.LocalDate instance. This is because there’s no suitable constructor or factory method present for this operation.”
The error implies that while processing the /room/reservation/v1 path, something went awry. This was particularly noted in the checkin field of the com.xxxxx.xxxxx.model.request.ReservationRequest object. The response status, unfortunately, was 400—indicating a “Bad Request”.
Dissecting the POST Request
The POST request being sent looks like this:
{
"id": 12345,
"checkin": "2018-12-14",
"checkout": "2018-12-17"
}
The request is a simple JSON object that includes an id and two dates – checkin and checkout. These dates are provided as strings in typical year-month-day format.
Understanding the Class Definitions
The ApiConfig class is a configuration class that sets up the applications ObjectMapper and ObjectWriter beans:
@Configuration
public class ApiConfig {
@Bean
public ObjectMapper objectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.registerModule(new JavaTimeModule());
return new ObjectMapper();
}
@Bean
public ObjectWriter objectWriter(ObjectMapper objectMapper) {
return objectMapper.writerWithDefaultPrettyPrinter();
}
}
The ObjectMapper is a vital piece of the puzzle. It is a part of the Jackson library that provides functionality for reading and writing JSON. The ObjectWriter, on the other hand, is a tool used for converting complex data structures to JSON.
The config class sets up an ObjectMapper that has the JavaTimeModule registered. This module extends the Jackson library’s functionality by adding support for Java 8’s new date and time API.
Next, we have the ReservationRequest class:
public class ReservationRequest {
private Long id;
@DateTimeFormat(iso = DateTimeFormat.ISO.DATE)
private LocalDate checkin;
@DateTimeFormat(iso = DateTimeFormat.ISO.DATE)
private LocalDate checkout;
public ReservationRequest() {
super();
}
public ReservationRequest(Long id, LocalDate checkin, LocalDate checkout) {
super();
this.id = id;
this.checkin = checkin;
this.checkout = checkout;
}
// getters and setters omitted for brevity
}
This class is a simple POJO (Plain Old Java Object) representing a reservation request. It has two fields of type LocalDate – checkin and checkout, which are annotated with @DateTimeFormat. This annotation specifies the format to use when parsing dates from a string.
The ReservationRequest class has a no-arg constructor and a full-arg constructor. The full-arg constructor allows creating a new ReservationRequest instance with all its fields specified.
Conclusion
In conclusion, the error messages displayed from both STS and Postman, alongside the utilization of relevant classes with their respective imports in the POST request formulation, suggest a meticulous approach to debugging. However, the suspicion surrounding potential oversights in constructors and annotations indicates the need for a thorough review of the codebase. The user’s encounter with an error while transmitting a JSON request through Postman underscores the importance of meticulous attention to detail in API development. Thus, a comprehensive investigation into the root cause of the issue is warranted to ensure smooth operation and reliability in future endeavors.