Unlock insights into troubleshooting the perplexing error message “React refers to a UMD global, but the current file is a module” through our comprehensive manual, enriched with invaluable remedies, snippets of code, and pertinent references. As a practitioner of React, encountering the aforementioned error message might present a formidable challenge, especially for novices navigating the realms of web development or React itself. Within this discourse, we shall delve into the essence of this error and its origins, while furnishing an array of solutions aimed at its resolution.
Deciphering the ‘React Refers to a UMD Global’ Error
Before delving into the troubleshooting process, it’s essential to grasp the concepts of ‘UMD Global’ and ‘Module’ thoroughly. These terms are fundamental in JavaScript development, especially when dealing with libraries like React.
Understanding UMD Global and Module:
- UMD Global: A UMD (Universal Module Definition) global is a versatile script designed to seamlessly operate in both server and browser environments. It offers adaptability and flexibility, making it a preferred choice for developers working across different platforms;
- Module: In the context of JavaScript, a module refers to a file containing reusable chunks of code that can be imported into other files. This modular approach enhances code organization, reusability, and maintainability.
Now, let’s explore the common issue that arises when dealing with React libraries and how to address it effectively.
Identifying the Problem:
When React library is imported as a standard JavaScript module instead of being treated as a UMD global, it often leads to errors. This mismatch in import approach can cause conflicts within the codebase, resulting in issues like the error message: “React refers to a UMD global, but the current file is a module. Consider adding an import instead.”
Tips and Recommendations for Resolution:
- Understanding the Difference: Gain a comprehensive understanding of the disparities between UMD Globals and modules. This foundational knowledge empowers you to troubleshoot similar issues in the future with confidence;
- Proper Import Usage: Always ensure that you are using the correct import statements for React or ReactDOM in your application. Incorrect import usage can introduce unexpected bugs and hinder the smooth functioning of your codebase;
- External Resource Reference: If your application relies on external resources, verify that they are not conflicting with your code. Sometimes, external dependencies can inadvertently interfere with the intended operation of your React components.
Deconstructing the Error
Before we delve into potential solutions, it’s paramount to grasp the distinction between a UMD global and a module. A UMD (Universal Module Definition) global is an adaptable script designed to run in both browser and server environments. It’s a popular and effective way to ensure that your JavaScript libraries are compatible across different environments.
On the other hand, a module in JavaScript serves as a container for reusable chunks of code, which can be exported and imported for use in other JavaScript files. This system allows developers to write clean and maintainable code by splitting complex programs into smaller, manageable parts.
The mentioned error tends to occur when React, which is designed to operate as a UMD global, is imported like a regular JavaScript module. This break in conventions leads your browser’s console to display the vexing error message: “React refers to a UMD global, but the current file is a module. Consider adding an import for this module.”
Understanding this error message is the first step towards its resolution. The problem lies with the method used to import the React and ReactDOM libraries into your code.
Recommendations For Understanding the Error:
- Clear distinction: Differentiate UMD Global and Modules thoroughly. Having a good hold on individual characteristics helps in identifying and rectifying errors better;
- Import Statements: Pay heed to the way React and ReactDOM are being imported in your code. A minor misstep here can lead to the error at hand;
- External Interference: Evaluate the impact of any external resources on your code. For instance, if you’re utilizing CDN for React and ReactDOM, it might conflict with your local module imports.
Integrating the Correct Import Statement
An immediate and effective way to rectify this error hinges on adding the correct import statement for React in your code. This statement needs to be inserted at the very beginning of your JavaScript file.
The basic syntax for importing React and ReactDOM in your JavaScript file is as follows:
import React from 'react';
import ReactDOM from 'react-dom';
Subsequently, you can utilize ReactDOM’s ‘.render’ method to input React components into the DOM:
ReactDOM.render(
<h1>Hello, world!</h1>,
document.getElementById('root')
);
This solution rectifies the error for most developers. However, there might be instances where this might not suffice due to the inherent complexities in your codebase or project structure.
Tips For Correct Import Statement:
- Understand the Syntax: Comprehend the basic syntax and usage of import statement in JavaScript;
- Proper Import Position: Always place the import statements at the beginning of your file;
- Debugging: Learn to debug effectively in case the default solution doesn’t work;
- Project Structure: Sometimes, the issue might be due to the specific project structure or configurations. Always verify these to identify any deviations.
Diving into Unique Scenarios Leading to the Error
Outside of the common reasons for this error, a few distinct scenarios can also lead to the “React refers to a UMD global” error. Understanding these unique situations enables you to tackle the error more effectively.
Dealing with Jest Tests
Should you encounter this error while running tests with Jest, a tweak in your Jest configuration file can rectify it. The process involves mapping the modules in a certain way to their respective paths. Insert the following lines in your Jest configuration to resolve the issue:
"moduleNameMapper": {
"^react$": "/node_modules/react",
"^react-dom$": "/node_modules/react-dom"
},
This piece of code ensures that your Jest tests correctly identify and utilize the React and ReactDOM modules.
Resolving TypeScript Errors
When working with TypeScript and the ‘@types/react’ package, it’s possible to come across a similar error. To troubleshoot this, add the following piece of code in your ‘tsconfig.json’ file:
"compilerOptions": {
"types": ["react", "react-dom"]
}
With this code in place, the TypeScript compiler will recognize ‘react’ and ‘react-dom’ by their @types packages.
Working with Chakra Modal
If you’re using a UI library like Chakra and its Modal component, you might encounter this error. To circumvent it, make sure to import the Modal component correctly:
import { Modal } from '@chakra-ui/react';
Refreshing the Development Server and IDE
Lastly, restarting the development server and Integrated Development Environment (IDE) often works wonders and resolves such inconsistencies. It’s a simple yet effective approach that deals with potential memory leaks or caching issues, if any.
Pro Tips for Unique Scenarios:
- Understand Jest Configurations: Familiarize yourself with Jest to understand how its configurations can affect your application;
- Explore TypeScript CompilerOptions: Know your TypeScript compiler options well for smooth and error-free coding sessions;
- Correct Component Import: Be careful while importing components from libraries like Chakra UI. A small error could lead to unwanted issues;
- Refreshing Environment: When in doubt, restarting the development server and IDE is a worthy trial. Many times, this simple act solves many hidden complex issues.
Exploring Additional Resolutions
While the aforementioned solutions typically rectify the “React refers to a UMD global” error, there are instances where you might need to explore other alternatives. Here are a few more tricks you can employ if the previously listed solutions aren’t working:
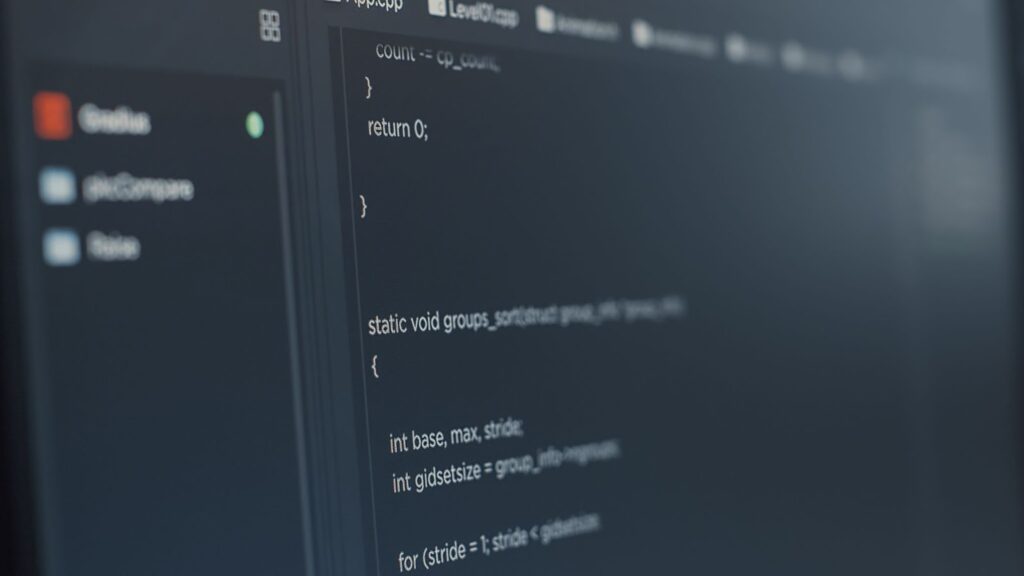
Updating JSX in Compiler Options
Modifying your configuration file to replace the JSX line with ‘jsx: “react-jsx”’ could potentially resolve the error. Here’s a sample illustration of how to go about it:
{
"compilerOptions": {
"jsx": "react-jsx"
}
}
This change informs the compiler to interpret JSX codes in the form supported by the most recent version of React, thereby helping to prevent errors associated with older JSX syntaxes.
Defining Externals in Webpack
Another avenue to explore is to define external modules as global variables in your webpack configuration. Essentially, this treats the modules not as parts of your bundle, but as global variables. Here’s a snippet depicting this approach:
// webpack.config.js
module.exports = {
externals: {
react: 'React',
'react-dom': 'ReactDOM'
}
};
In the context of this error, defining React and ReactDOM as externals ensures your code doesn’t confuse the UMD global React and ReactDOM with their module counterparts.
Utilizing SVG Files as Components
The error might also occur when you’re attempting to use SVG files as components imported from another source. If this is the case, make sure you’re importing and using SVGs correctly within your React components. Check out the code snippet below for better understanding:
import { ReactComponent as Logo } from './logo.svg';
function App() {
return (
<div>
<Logo />
</div>
);
}
In this example, the SVG logo is imported as a React component and then used in the App component.
Tips to Further Troubleshoot the Error:
- Explore Compiler Options: If the typical solutions aren’t working, look into your compiler options. A minor modification there can sometimes do the trick;
- Understand Webpack Externals: Understanding how to work with webpack externals is crucial in resolving module conflicts in your bundle;
- SVG as Components: Ensure you are correctly importing and using SVGs in your React components.
Keeping Up with Recent React Changes
With the advent of React 17, one significant modification is that developers no longer have to explicitly import React from “react”. This tweak simplifies the process of utilizing React in your projects, but it can also give rise to certain issues. Particularly, this deviation from established norms can trigger unforeseen errors.
Moreover, it’s important to note that this change may cause problems with other libraries that still expect the “import React” statement. Hence, developers need to remain vigilant and adapt their coding practices accordingly.
‘React Refers to a UMD Global’ Error: Additional JavaScript Examples
The crux of the issue lies with UMD globals and their compatibility with the current module file. Traditionally, the solution is to prefer import statements over directly using UMD globals.
Consider the following JavaScript code snippet to understand better:
import React from ‘react’;
By importing React in this manner, you sidestep the risk of treating it as a UMD global that doesn’t fit into the current module file.
Tips for Navigating React’s Latest Developments:
- Updated Import Practices: With React 17, adjust your coding habits to reflect the changes involving the import of React;
- Library Compatibility: Be aware that certain libraries might still expect the traditional “import React” statement and could throw errors if it’s missing;
- Know Your UMD Globals: Understanding UMD globals and their role in the context of React helps in dealing with similar issues efficiently;
- Prioritize Import Statements: Favor the usage of import statements instead of invoking UMD globals directly in your code. This practice offers better clarity and helps avoid potential errors.
Conclusion
In conclusion, armed with a deeper understanding of the error message “React refers to a UMD global, but the current file is a module,” React developers are now equipped to navigate and overcome this challenge with confidence. By leveraging the insights provided in our comprehensive guide, developers can efficiently troubleshoot and resolve this issue, ensuring smooth progression in their web development endeavors. With a wealth of solutions, code snippets, and relevant resources at their disposal, developers can tackle this error head-on, ultimately enhancing their proficiency and mastery of React.