When employing let statements within case blocks within a reducer, ESLint raises a warning. To circumvent this issue, it is advisable to opt for a block instead, thereby confining the variable’s scope solely to the particular block in question, effectively mitigating ESLint warnings.
Understanding Unexpected Lexical Declaration in Case Block
While working with reducers in JavaScript, there might be scenarios where modification of state is required. For instance, consider the following situation, where the task is to delete an interest of the user:
case DELETE_INTEREST:
let deleteInterests = state.user.interests;
let index = deleteInterests.findIndex(i => i == action.payload);
deleteInterests.splice(index, 1);
return { ...state, user: { ...state.user, interests: deleteInterests } };
However, ESLint, a popular JavaScript linting tool, may flag the usage of let statements within reducer case blocks. This happens due to the ‘no-case-declaration’ rule, which detects an unexpected lexical declaration within a case block.
Why Does ESLint Discourage Declaring Variables Within Case Blocks?
The reason for this flagging is that using let statements inside case blocks can cause the variable to remain in scope beyond the current case. However, confining such statements within a block ensures the variable’s scope is limited to that specific block only.
For this purpose, curly brackets {} can be used to create a block scope for the case, as shown below:
case DELETE_INTEREST: {
let .....
return (...)
}
Consider the following examples to gain a better understanding:
function withOutBraces() {
switch(1) {
case 1:
let a=10;
console.log('case 1', a);
case 2:
console.log('case 2', a);
}
}
function withBraces() {
switch(1) {
case 1: {
let a=10;
console.log('case 1', a);
}
case 2: {
console.log('case 2', a);
}
}
}
console.log('========First Case ============')
withOutBraces()
console.log('========Second Case ============')
withBraces();
Alternative Approach to Modifying Arrays
To remove an element from an array without modifying the original, an alternative approach is to use the array.filter method instead of splice. The reasoning behind this is that the filter method returns a new array, thus reducing the risk of unintentionally altering the original array. The revised code would be as follows:
case DELETE_INTEREST:
let deleteInterests = state.user.interests;
let newData = deleteInterests.filter(i => i !== action.payload);
return { ...state, user: { ...state.user, interests: newData } };
This code snippet provides the same functionality, but with enhanced scoping and array management, optimizing for both understanding and performance.
Adopting a Protective Approach with the Curly Braces ‘{}’
Sometimes, you might be dealing with a scenario where you’ll have to put all the operations inside a case statement for a reducer. Including these operations within braces {} offers a structured and safer way to execute. This method effectively encases the operations, containing them within the case block. Let’s examine this approach via an uncomplicated sample.
Sample Interaction
For simplifying the concept, let’s consider we’re dealing with an online shopping cart where an item needs to be removed. This action could be represented, for instance, as EnumCartAction.DELETE_ITEM.
The case block would look like this:
case EnumCartAction.DELETE_ITEM: {
const updatedItems = state.cart.filter((item) => item._id !== action.payload);
return {
...state,
cart: updatedItems
};
}
Here, all the contents are enclosed within the curly braces {}. This technique encapsulates each operation inside the case statement, making sure there’s no unexpected scope leakage.
In-Depth Explanation and Best Practices
In the code snippet above:
- EnumCartAction.DELETE_ITEM is the action type that triggers the removal of a specific item from the cart;
- The filter() method is used to create a new array updatedItems, which includes all the items in the current state’s cart, except for the one to be deleted. This is determined by the condition item._id !== action.payload;
- A new state is then returned with the updated cart.
This way of writing the code allows for scope isolation and better code readability and organization. It’s a best practice worth adopting to make your code cleaner, easier to debug, and more maintainable in the long run.
Remember, for optimal coding practices:
- Always encapsulate your case contents within curly braces {} to effectively maintain the scope;
- Use the filter() method for non-destructive array manipulation, keeping your original data intact while making modifications.
Following these principles will assist in avoiding unexpected outcomes, ensuring your application behaves as intended. They will also keep your code well-structured and easy to understand, making it easier for you and others to work with.
Unraveling the “Can’t Access Lexical Declaration Before Initialization” Error
As developers, encountering error messages is part of our daily grind. One such error message that might appear reads: “Can’t access lexical declaration X before initialization”. When this pops up, it means the program is trying to access a variable before it has been defined in the code. This issue typically arises when using let and const declarations inside any block statement.
The Error Message
Depending on the browser used, this error can manifest in a few different ways:
- Microsoft Edge: ReferenceError: Can’t access ‘X’ before initialization;
- Firefox: ReferenceError: Can’t access lexical declaration ‘X’ before initialization;
- Chrome: ReferenceError: ‘X’ is not defined.
Despite the slight differences in wording, all these error messages point to the same underlying problem: premature access to a variable.
Understanding the Problem
Variables declared using let and const are hoisted to the top of their scope – that is, the JavaScript engine moves the declarations to the top of their enclosing scope during the compilation phase. However, unlike var declarations, these variables are not initialized in the process. Instead, they remain in a “temporal dead zone” (TDZ) until execution reaches their point of declaration and they’re finally initialized.
If an attempt is made to access these variables while they’re still in the TDZ, the JavaScript engine throws a ReferenceError, indicating that the variable can’t be accessed before initialization.
This behavior ensures const variables cannot be accessed or assigned until they’ve been initialized, reinforcing their constant nature.
Prudent Coding Practices to Avoid This Error
Here are a few tips to help dodge this error:
- Always initialize variables at the time of declaration;
- Avoid using a variable before it’s declared and initialized;
- Understand the scoping rules and how hoisting works in JavaScript.
Building an understanding and following these practices will help you to create more reliable and bug-free code.
Walking through ‘Can’t Access Lexical Declaration X before Initialization’
When developing with JavaScript, you may come across an error message stating, “Can’t access lexical declaration X before initialization”. This can be a bit mystifying for beginners, and even experienced coders can be thrown off their stride by this error, especially when the coding gets complex.
Diving into the Error Message
This error message manifests itself in different forms depending on the web browser in use:
- Edge: ReferenceError: Can’t access ‘X’ before initialization;
- Firefox: ReferenceError: Can’t access lexical declaration ‘X’ before initialization;
- Chrome: ReferenceError: ‘X’ is not defined.
In essence, they all point to the same issue: trying to access a let or const variable before it has been defined or initialized in the code block. This highlights one of the key differences between var and let/const declaration types.
Breaking Down the Problem
A JavaScript variable declared with let or const does not become accessible until the code execution reaches its declaration. Prior to that, any attempt to call or manipulate the variable will result in the error described above. This is because let and const have block scope and are not hoisted, unlike var variables.
Troublesome Example
Here is an instance of this problem:
function exampleFunction() {
// Trying to log 'foo' before it's declared
console.log(foo); // ReferenceError: Can't access lexical declaration 'foo' before initialization
let foo = 33;
}
exampleFunction();
In the function above, the variable foo is accessed before it’s declared with let, causing a ReferenceError.
The Correct Approach
Here is a corrected version of the previous function:
function exampleFunction() {
// Declaring the variable 'foo' before trying to access it
let foo = 33;
console.log(foo); // Logs '33' to the console
var bar = 12;
console.log(bar); // Logs '12' to the console
}
exampleFunction();
In this function, the variable foo is declared before it’s accessed, preventing any ReferenceError. The variable bar is also declared with var before being logged, preventing any errors even though var variables are hoisted and can be accessed before their declaration.
Potential Pitfalls and Recommendations
To avoid this error:
- Always declare let and const variables before attempting to access them;
- Understand the difference in scoping and hoisting between var, let, and const.
By following these tips and understanding the underlying concepts, you can write cleaner, error-free JavaScript code.
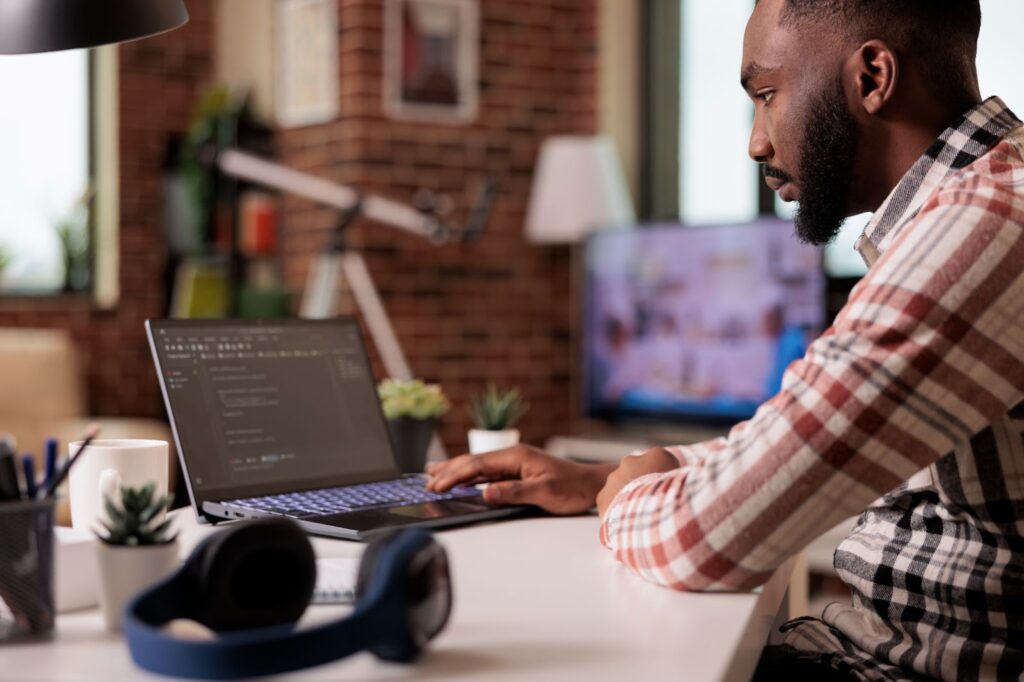
Resolving the Syntax Error: Lexical Declaration Cannot Appear in a Single-Statement Context
Embarking on the journey of creating your first discord bot may throw some unexpected errors at you. One such stumbling block is the syntax error saying: “Lexical declaration cannot appear in a single-statement context”. Although it may sound daunting, let’s simplify this error and the steps to solve it.
An Overview of the Problem
The sample code provided looks like a typical discord bot setup. There are various recognizable elements, such as defining constants, setting up the bot client, and having the bot react to messages. But there’s a line of code that throws an error:
else if (command == 'play') let args = message.content.substring(prefix.length).split(" ");
This let keyword and the subsequent declaration are causing the error. The issue is that a let declaration cannot appear directly after an if or else if statement, without being enclosed in curly braces {}. This is a syntax requirement in JavaScript.
Solving the Problem
A simple fix to this problem is to enclose the let statement and the subsequent logic within curly braces {}. Here’s an example of how to correct the issue:
else if (command == 'play')
{
let args = message.content.substring(prefix.length).split(" ");
// Rest of the code here
}
Now, the let statement is enclosed in its own block scope and the syntax error should no longer appear.
When creating a discord bot, it’s essential to adhere to correct JavaScript syntax to avoid errors that halt the bot’s operation. By learning from these common issues, we can write cleaner, more efficient code and create a bot that interacts seamlessly with your Discord community. Always remember that:
- Syntax is crucial in JavaScript. Make sure to follow correct grammar rules, such as enclosing block statements within curly braces {};
- Reading and understanding error messages can provide significant insight into what’s going wrong in your code.
Deep Dive into Understanding the Unexpected Lexical Declarations in Case Blocks
In JavaScript programming, there are occasions when coders encounter obstacles in defining variables using the let keyword, particularly within a context requiring a singular statement. For instance:
} else if (command == 'play')
let args = message.content.substring(prefix.length).split(" ");
In this example, the let variable args is incorrectly defined. This is because the let declaration is expected to be within a block scope, and not in a single-statement context.
To mitigate this issue, coders can create a multi-statement context by enclosing the code within a set of curly brackets {}. This practice is essential as it provides the correct scope for the let keyword.
} else if (command == 'play') {
let args = message.content.substring(prefix.length).split(' ');
// ...
}
In this revised code snippet, you’ll notice that the let statement is now inside a block scope, made possible by the enclosing curly brackets.
During a discussion on eslint: no-case-declaration – unexpected lexical declaration in case block on June 8, 2018, user Mayank Shukla highlighted this issue. In fact, developers frequently encounter this quirk of JavaScript variable declaration. Other relatable scenarios include:
- Passing an object as props to JSX (Discussed on March 3, 2018);
- Implementing if-else statements within JSX for ReactJS (Posted on May 18, 2017);
- Embedding if conditions inside the map() function in React (A discussion held on July 7, 2017);
- Acquiring the TextField value when the enter key was pressed.
All these examples illustrate the importance of using correct syntax to define variables in JavaScript, especially when dealing with case blocks and the let keyword. Understanding the scope context can significantly enhance the execution of your JavaScript code and prevent error messages related to unexpected lexical declarations.
Understanding Block Scoping in ES6 Switch-Case Statements
Are you puzzled by block scoping in ES6 switch-case statements? Let’s inspect this fascinating JavaScript feature more closely.
Look at this code:
switch (false) {
case true:
let x = "bar";
console.log(x);
break;
case false:
let x = "baz";
console.log(x);
break;
}
Here, the switch-case statement attempts to declare the variable x twice within the same block scope. If you’re familiar with ECMAScript 2015 (ES6), you’ll know that this is not permissible. In ES6, multiple declarations of the same identifier within a single scope are not allowed. Because of this, the code above throws an error.
The Magic of Curly Braces
Curly braces aren’t just a stylistic choice in JavaScript; they have a distinct functionality. When you use curly braces ({}) in a switch-case statement, you create a new block scope. Block scopes allow you to declare local variables or constants with let and const.
switch (false) {
case true: {
let x = "bar";
console.log(x);
break;
}
case false: {
let x = "baz";
console.log(x);
break;
}
}
In the revised code above, each case now has its own block. This allows the variable x to be declared twice without causing an error. Each declaration of x exists within its own block and does not conflict with the other.
It’s crucial to note that a switch statement itself forms a block scope. Any let or const declarations made within this switch will not leak into the parent scope, regardless of whether nested block scopes are used or not. Therefore, curly braces serve to enhance readability by delineating the blocks of individual case branches.
Mastering Curly Braces in JavaScript: Block Scoping and Syntax Tips
Are you puzzling over curly braces in JavaScript? It’s time to unlock their potential! In JS, curly braces { } create a distinct block scope: a space where you can declare local variables and constants using let and const. Here’s an example:
switch (false) {
case true: {
let x = "bar";
console.log(x);
break;
}
case false: {
let x = "baz";
console.log(x);
break;
}
}
In ECMAScript 2015 (ES6), a single scope cannot contain multiple declarations with the same identifier. Therefore, nested block scopes (created by curly braces) are crucial to prevent conflicts between let and const declarations.
Note-Worthy Points and Best Practices
- Block Scope of Switch Statements: A switch statement naturally forms a block scope. Therefore, any let or const declarations inside the switch won’t leak into the parent scope, even without nested block scopes;
- Visual Enhancement: The use of curly braces clearly demarcates individual case branches for enhanced readability. Always consider this when writing a switch statement.
Now, let’s tackle some common errors and their solutions:
If you accidentally declare the same variable twice within the same block scope, you’ll meet an Uncaught SyntaxError, as it’s against ES6 rules to do so. For instance:
let variablename = "test";
let variablename = "newTest"; //SyntaxError: Identifier 'variablename' has already been declared
To remedy this, uniquely name all variables within a single block scope to steer clear of redundancies and ensure smooth code execution.
ESLint, a popular JavaScript linter, also catches errors related to block scoping in switch statements. If you declare a let or const variable within a case without using curly braces, you’ll encounter the Unexpected lexical declaration in case block no-case-declarations error.
Check this example:
function withoutBraces() {
switch (1) {
case 1:
let a = 10;
console.log('case 1', a);
case 2:
console.log('case 2', a)
}
}
This code will throw an error because of the let declaration within the case branch. Simply add curly braces {} to create a new block scope:
function withBraces() {
switch (1) {
case 1: {
let a = 10;
console.log('case 1', a);
}
case 2: {
console.log('case 2', a)
}
}
}
Now the code will execute flawlessly!
In conclusion, understanding and applying block scoping effectively in JavaScript can significantly impact your code’s readability and functionality. So don’t overlook those curly braces, they’re more powerful than they seem!
Conclusion
In conclusion, opting for a block instead of using let statements within case blocks in a reducer is a recommended approach to address ESLint warnings. By confining the variable’s scope to the specific block, potential issues can be effectively managed, ensuring cleaner and more compliant code.