You can discover a functional code snippet that fulfills the requirement of throwing a checked exception, distinct from an unchecked one, by employing a particular method. Achieving a comparable outcome using plain Mockito necessitates employing the suitable method. Alternatively, employing Kotlin and the “given” function offers another avenue for resolution. It is noteworthy that Mockito does accommodate checked exceptions provided they are declared in the method signature, exemplified in a demonstration. Nonetheless, if a checked exception is thrown without being declared in the method signature, Mockito will falter, yielding a generic error message that might mislead. This discrepancy does not imply a general lack of support for checked exceptions but rather indicates that the specific exception thrown is inappropriate for that method.
Resolving the “Checked Exception is Invalid for this Method” Error in Mockito
In the world of Java programming, encountering the error “Checked exception is invalid for this method!” when using Mockito can be a hassle. This error typically occurs when the Mockito library is leveraged to create a mock object for testing.
Understanding the Issue
Let’s examine a common scenario. While running a test method, there is a line of code that fails and presents the “Checked exception is invalid for this method!” error message. This scenario occurs when an attempt to use a mock object is made, as illustrated below:
InitialContext mockContext;
mockContext = mock(InitialContext.class);
when((DataSource) mockContext.lookup("java:comp/env/jdbc/foo")).thenThrow(new ConnectionFactoryException("test")); // <-- Fails on this line
The Test Method in Question
The @Test method shouldThrowExceptionIfDataSourceDoesNotExist() is written to verify if a ConnectionFactoryException is thrown when the DataSource does not exist. The code snippet is as follows:
@Test
public void shouldThrowExceptionIfDataSourceDoesNotExist() throws ConnectionFactoryException {
assertThatExceptionOfType(ConnectionFactoryException.class)
.isThrownBy(() -> { new DataSourceFactory(mockContext).getDataSource("foo"); })
.withMessage("Unable to find jdbc/foo");
}
The Class Undergoing Test
The class being tested is the DataSourceFactory class that has dependencies on the InitialContext class. This class includes a method getDataSource(String dataSourceName), which is expected to throw a ConnectionFactoryException when it is unable to locate the appropriate DataSource.
public class DataSourceFactory {
// Dependencies to be injected
private InitialContext context;
public DataSourceFactory(InitialContext context) throws ConnectionFactoryException {
if(context == null) {
throw new ConnectionFactoryException("context can't be null");
}
this.context = context;
}
public DataSource getDataSource(String dataSourceName) throws ConnectionFactoryException {
if(dataSourceName == null) {
throw new ConnectionFactoryException("dataSourceName can't be null");
}
// Ensure we have the correct syntax for the datasource name
if(!dataSourceName.startsWith("jdbc/")) {
dataSourceName = "jdbc/" + dataSourceName;
}
try {
System.out.println("java:comp/env/" + dataSourceName);
DataSource dataSource = (DataSource) context.lookup("java:comp/env/" + dataSourceName);
return dataSource;
} catch (NamingException e) {
throw new ConnectionFactoryException("Unable to find " + dataSourceName, e);
}
}
}
Although it’s normally advised to avoid mocking objects that aren’t owned by the class under test, it is challenging to find an alternative approach to test the efficacy of the getDataSource() function without resorting to mocking the InitialContext object.
Ways to forge ahead
Testing methods that interact with external components like a database can be challenging. However, several approaches can be considered to resolve the “Checked exception is invalid for this method!” error:
- Utilizing an Embedded Database: This could serve as a lightweight, rule-based in-memory database for testing;
- Leveraging Interfaces: Another alternative could be defining an interface for the data source retrieval operation and providing different implementations for test and production;
- Using a Test Double: A stub or a fake could be used to simulate the behavior of the external component.
It’s imperative to select an approach that aligns best with the testing objectives and the nature of the application. Exploring these options will foster a better understanding of their applicability and effectiveness, thereby enhancing Java programming proficiency.
Unraveling the ‘Mockito Checked Exception is Invalid for this Method’ Conundrum
Identifying the Error
While delving into Java programming, it’s often a roadblock when a mock attempt triggers an error that reads ‘Mockito Checked Exception is Invalid for this Method’. Often developers encounter this hiccup when they employ Mockito to create a mock object for testing.
Consider the following code snippet:
InitialContext mockContext;
mockContext = mock(InitialContext.class);
when((DataSource) mockContext.lookup("java:comp/env/jdbc/foo")).thenThrow(new ConnectionFactoryException("test")); // <-- Error occurs here
In the code, the error arises during the execution of a test method that is supposed to throw a ‘ConnectionFactoryException’ if it cannot locate a specific ‘DataSource’:
@Test
public void shouldThrowExceptionIfDataSourceDoesNotExist() throws ConnectionFactoryException {
assertThatExceptionOfType(ConnectionFactoryException.class)
.isThrownBy(() -> { new DataSourceFactory(mockContext).getDataSource("foo"); })
.withMessage("Unable to find jdbc/foo");
}
The Class in Focus
The class under the microscope here is DataSourceFactory. It depends on the InitialContext class and contains a method getDataSource(String dataSourceName). This method, in turn, is expected to throw a ConnectionFactoryException if it fails to track down the relevant DataSource. Here’s how the class looks:
public class DataSourceFactory {
// Dependencies to be injected
private InitialContext context;
public DataSourceFactory(InitialContext context) throws ConnectionFactoryException {
if(context == null) {
throw new ConnectionFactoryException("context can't be null");
}
this.context = context;
}
public DataSource getDataSource(String dataSourceName) throws ConnectionFactoryException {
if(dataSourceName == null) {
throw new ConnectionFactoryException("dataSourceName can't be null");
}
// Ensure we have the correct syntax for the datasource name
if(!dataSourceName.startsWith("jdbc/")) {
dataSourceName = "jdbc/" + dataSourceName;
}
try {
System.out.println("java:comp/env/" + dataSourceName);
DataSource dataSource = (DataSource) context.lookup("java:comp/env/" + dataSourceName);
return dataSource;
} catch (NamingException e) {
throw new ConnectionFactoryException("Unable to find " + dataSourceName, e);
}
}
}
While it is generally discouraged to work with mock objects that are beyond a developer’s control, the testing efficiency of the getDataSource() function seems heavily dependent on the mock InitialContext object.
Navigating through the Issue
Writing tests for methods that interact with components outside of the application, such as databases, can be tricky. Still, there are several routes to explore to bypass the ‘Mockito Checked Exception is Invalid for this Method’ error:
- Using an Embedded Database: This could be used as a transient, rules-based in-memory database pertinent for testing;
- Harnessing Interfaces: An interface can be engineered for the data source retrieval operation and different implementations could be provided for testing and production;
- Employing a Test Double: A stub or a fake could imitate the behavior of the foreign element.
Developers must adopt a pathway that syncs with their testing goals, as well as the essence of the application. Investigating these alternatives will not only resolve the problem at hand, but also enrich the understanding of their utility and efficiency, thereby amplifying Java programming savvy.
Overcoming the Hurdle: Solutions & Suggestions
Rectifying the Error
In the case of the code triggering an incorrect exception, it’s pivotal to realize that the lookup does not actually trigger a ConnectionFactoryException. So, to ensure the code handles the given situation correctly, it is suggested to throw the right exception – the NamingException. Following which, testing can be done to verify if the code properly addresses this exception by ensuring it throws a ConnectionFactoryException.
Here’s how the code modification looks like:
when(mockContext.lookup("java:comp/env/jdbc/foo"))
.thenThrow(new NamingException("test"));
Understanding Checked and Unchecked Exceptions
In Java, exceptions are categorized into checked and unchecked. The Java API defines several exception classes that are either checked or unchecked. Additionally, developers are free to declare their own exception classes, both checked and unchecked.
Here are some quick tips on how to identify checked and unchecked exceptions:
- Checked Exceptions: These are the exceptions that a method can throw, as indicated by the throws keyword in the method declaration. Since the compiler checks these exceptions at compile-time, they are known as checked exceptions;
- Unchecked Exceptions: These are exceptions that are not declared in the method signature. The compiler does not check these exceptions at compile-time, hence why they are called unchecked exceptions.
Remember, it is always possible to ascertain the type of exception at runtime using instanceof, although it’s not generally recommended.
Understanding these distinctions can help developers navigate exception handling more smoothly, thereby making Java programming a more seamless affair.
Conquering the ‘MockitoException: Checked Exception is Invalid for this Method’ Challenge in JUnit5 and Spring Boot Testing
While performing service layer testing using JUnit5 and Spring Boot 2.6.2, it’s crucial to validate whether an exception appears when an account is not found. The goal is to ensure that the system behaves as expected even in an unfavorable circumstance.
Below is a test method written to inspect this aspect of the functionality. However, the test encounters a ‘MockitoException: Checked Exception is Invalid for this Method’ error:
@Test
void getUserAccountWithUserAccountNotFoundExceptionTest() {
/**
* TODO tried to test it but getting this error
* Checked exception is invalid for this method
*/
when(this.systemUserRepository.findByEmailAddress(emailAddress)).thenThrow(UserAccountNotFoundException.class);
Assertions.assertThrows(UserAccountNotFoundException.class, ()->{
this.manageUserAccountService.getUserAccount(emailAddress);
});
}
To understand the scenario, let’s examine the related service implementation for the ‘ManageUserAccountServiceImpl’ class. The ‘getUserAccount’ method is overridden here to retrieve a ‘UserAccountDto’ object based on the user’s email address. In cases where the user account is not found, a ‘UserAccountNotFoundException’ is thrown.
To handle this exception, a ‘UserAccountNotFoundException’ class is created, as shown below. It extends the ‘UserAccountException’ class and involves a mix of constructors to handle multiple scenarios:
public class UserAccountNotFoundException extends UserAccountException {
public UserAccountNotFoundException() {
super();
}
public UserAccountNotFoundException(int errorCode, String errorMessage) {
super(errorCode, errorMessage);
}
public UserAccountNotFoundException(Throwable cause, boolean enableSuppression, boolean writableStackTrace,
int errorCode, String errorMessage) {
super(cause, enableSuppression, writableStackTrace, errorCode, errorMessage);
}
public UserAccountNotFoundException(Throwable cause, int errorCode, String errorMessage) {
super(cause, errorCode, errorMessage);
}
}
Recommendations and Solutions
Although Mockito is a powerful tool that can help simulate different situations, it’s important to use it correctly to prevent errors like ‘MockitoException: Checked Exception is Invalid for this Method’.
A crucial tip for the successful application of Mockito in this context is to ensure that you’re matching the correct exception types during unit testing. In other words, the exception thrown by the mock object should match the exception that the tested method is expected to throw.
Another recommendation is to use concrete instances of exceptions instead of class references when defining the behavior of mock objects. Providing a concrete instance of the exception instead of a class object as an argument to the ‘thenThrow()’ method ensures that Mockito can instantiate the exception correctly, avoiding errors.
For example, your test method can be revised as follows:
@Test
void getUserAccountWithUserAccountNotFoundExceptionTest() {
when(this.systemUserRepository.findByEmailAddress(emailAddress)).thenThrow(new UserAccountNotFoundException());
Assertions.assertThrows(UserAccountNotFoundException.class, () -> {
this.manageUserAccountService.getUserAccount(emailAddress);
});
}
By adopting these suggestions and meticulously employing Mockito, one can efficiently test the service layer and ensure it behaves as expected under a variety of scenarios.
Tackling the ‘MockitoException: Checked Exception is Invalid for this Method’ Hurdle with JUnit5 and Spring Boot 2.6.2
The Context
While implementing service layer testing using JUnit5 and Spring Boot 2.6.2, developers often come across a method that verifies an exception’s emergence when an account can’t be located. This verification ensures that the system continues to work as expected, even when an adverse scenario arises.
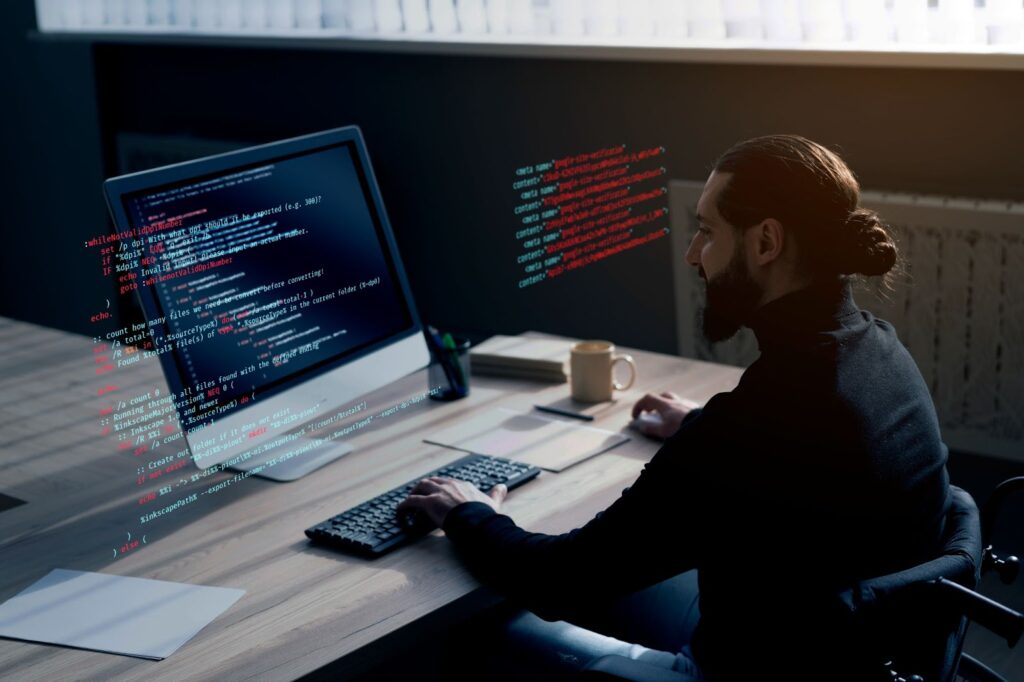
However, issues often surface while testing. Below is an example of a test method wherein the ‘MockitoException: Checked Exception is Invalid for this Method’ error makes an appearance:
@Test
void getUserAccountWithUserAccountNotFoundExceptionTest() {
/**
* TODO tried to to test it but getting this error
* Checked exception is invalid for this method
*/
when(this.systemUserRepository.findByEmailAddress(emailAddress)).thenThrow(UserAccountNotFoundException.class);
Assertions.assertThrows(UserAccountNotFoundException.class, () -> {
this.manageUserAccountService.getUserAccount(emailAddress);
});
}
The method’s role is to test the manageUserAccountService’s getUserAccount() method. It’s a function in the ManageUserAccountServiceImpl class where a UserAccountDto object is meant to be retrieved based on a provided email address. If the user account isn’t found, then a UserAccountNotFoundException is triggered.
The UserAccountNotFoundException Class
To manage the exception when a user account cannot be found, a class is established, named UserAccountNotFoundException. This class, a subset of the UserAccountException class, includes several constructors to manage various scenarios effectively:
public class UserAccountNotFoundException extends UserAccountException {
public UserAccountNotFoundException() { super(); }
public UserAccountNotFoundException(int errorCode, String errorMessage) { super(errorCode, errorMessage); }
public UserAccountNotFoundException(Throwable cause, boolean enableSuppression, boolean writableStackTrace, int errorCode, String errorMessage) {
super(cause, enableSuppression, writableStackTrace, errorCode, errorMessage);
}
public UserAccountNotFoundException(Throwable cause, int errorCode, String errorMessage) { super(cause, errorCode, errorMessage); }
}
The UserAccountException Class
The UserAccountException class, an extension of the standard Exception class, is a custom, user-defined exception that handles all user account-related issues. This exception class is highly versatile, thanks to a multitude of constructors for handling a variety of error scenarios:
public class UserAccountException extends Exception {
private static final long serialVersionUID = -591169136507677996L;
protected ErrorInfoDto errorInfoDto;
public UserAccountException() { super(); }
public UserAccountException(int errorCode, String errorMessage) { this.errorInfoDto = formErrorInfoDto(errorCode, errorMessage); }
public UserAccountException(Throwable cause, int errorCode, String errorMessage) { this.errorInfoDto = formErrorInfoDto(errorCode, errorMessage); }
public UserAccountException(Throwable cause, boolean enableSuppression, boolean writableStackTrace, int errorCode, String errorMessage) {
this.errorInfoDto = formErrorInfoDto(errorCode, errorMessage);
}
private ErrorInfoDto formErrorInfoDto(int errorCode, String errorMessage) {
ErrorInfoDto errorInfoDto = new ErrorInfoDto();
errorInfoDto.setErrorCode(errorCode);
errorInfoDto.setErrorMessage(errorMessage);
return errorInfoDto;
}
public ErrorInfoDto getErrorInfoDto() { return errorInfoDto; }
}
The SystemUserRepository Interface
In this system, the SystemUserRepository interface extends Spring Data JPA’s JpaRepository. It includes methods that count the number of email addresses and mobile numbers and find users by email:
public interface SystemUserRepository extends JpaRepository {
long countByEmailAddress(String emailAddress);
long countByMobileNo(String mobileNo);
Optional findByEmailAddress(String emailAddress);
}
This interface paves the way for cleaner, simpler code and reduces the need for boilerplate code. It provides methods for CRUD operations, pagination, and sorting while also enabling the creation of custom methods.
Understanding these pieces of the system and how they interact is crucial to solving the ‘Checked Exception is Invalid for this Method’ error in Mockito. In so doing, developers can maintain robust, error-free testing systems.
Conclusion
In conclusion, exploring various methods and approaches such as utilizing specific methods, plain Mockito, or leveraging Kotlin with the “given” function provides solutions for meeting requirements regarding checked exceptions. While Mockito supports checked exceptions when declared in method signatures, caution is necessary to prevent misleading errors when exceptions are not appropriately declared. This underscores the importance of understanding the nuances of exception handling in different contexts to ensure robust and error-free code implementation.