If you find yourself needing to update package declarations from “package abc;” to “package com.aa.bb.cc.abc;” across multiple files, Eclipse offers a convenient solution through its Search->File feature. Simply navigate to the desired folder, package, or even the entire workspace, and initiate the search for “package abc;”. Instead of opting for the Search function, select “Replace” and input “package com.aa.bb.cc.abc;” when prompted for the replacement after the search is complete. This streamlined process ensures efficient and accurate modification of package declarations throughout your project.
Understanding the ‘Must Declare A Named Package’ Error in Java
Java, being a highly versatile and widely used language, occasionally throws errors that may seem puzzling at first. One such error is ‘Must declare a named package’. This error often occurs when trying to access a program in a specific named module, causing frustration amongst developers.
Understanding and navigating this error is crucial for seamless coding experience in Java. This article aims to break down this error, its causes, and the potential solutions for it.
Dissecting the Error Message: The Problem
When attempting to access a previously created program in Java, it is not uncommon to encounter the following error:
Must declare a named package due to an issue with the compilation unit associated to the named module
This error message implies that the Java compiler expects a package declaration for the program. However, this package declaration may not have been made, resulting in an error. The module mentioned in this error message could be any module within the Java project.
Solution 1: Removing module-info.java File
The first solution to tackle this error is rather straightforward:
- Navigate to your Project Explorer in Eclipse (or respective IDE);
- Identify the module-info.java file;
- Delete this file.
The module-info.java file is generated by default when the project is created. While it may seem counter-intuitive, deleting this file will not impact the overall functionality of your program. It is safe to remove this file, which often resolves the error.
Solution 2: Transferring Error Classes to New Package
If the first solution does not work or if you’re looking for a different approach, there’s an alternate method that could do the trick. This involves creating a new package and transferring the classes from the default package to this newly created package.
Here are the steps to follow:
- Create a fresh package within your project;
- Identify the classes, which have been giving the error;
- Move these classes from your default package to this newly created package.
Be careful while moving the classes to ensure that no dependencies are broken in the process. Once the classes are successfully moved into the new package, the error should be resolved.
In conclusion, encountering the ‘Must declare a named package’ error can be a source of frustration, but with these solutions at hand, you can tackle it with ease and continue your smooth journey in Java programming.
Remember, understanding the language and getting to know its quirks is part of becoming a proficient Java developer. Happy coding!
The Dreaded ‘Must Declare a Named Package’ Error When Importing Scanner
While working with Java, an error message, “Must declare a named package because this compilation unit is associated to the named module”, is frequently encountered. This becomes particularly noticeable when attempting to import the scanner class in a Java program.
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
String sentence = keyboard.nextLine();
System.out.println(sentence);
}
}
This error message indicates that the current Java class file, including the “Main” class in this case, is located directly within the “project/src” directory. This setup contravenes Java’s structure and triggers the mentioned error, especially when dealing with modules. This issue usually occurs due to the presence of Main.class in the main directory, which modules do not permit without a named package.
How to Address the Error
To adhere to Java’s standard file structure, and hence resolve this error, a worthy approach entails creating a pertinent directory under the “project/src” folder and treating this directory as a package. To illustrate, if the package name is “stackoverflow”, then create the “project/src/stackoverflow” directory and relocate “Main.java” there. Also, at the commencement of “Main.java”, make sure to declare the package name.
This could translate into:
package stackoverflow;
import java.util.Scanner;
class Main {
public static void main(String[] args) {
Scanner keyboard = new Scanner(System.in);
String sentence = keyboard.nextLine();
System.out.println(sentence);
}
}
With this setup, the program respects Java’s structure, and the error won’t appear.
Furthermore, integrated development environments (IDEs) like Eclipse might also show this error if the declared package doesn’t match the actual file directory structure. In such cases:
- Verify that the package declaration at the top of your Java classes matches the directory structure;
- If it doesn’t, correct it to match the file path.
Hence, understanding and respecting the Java file and package structure can save developers from encountering unexpected errors, thereby ensuring a more productive coding experience.
How to Update Package Declarations across an Entire Project in Eclipse
While working on projects in Eclipse, one might encounter a scenario where a large amount of source code needs to be imported. Consequently, due to the code’s relocation into distinct directories, the initial package name may become inapplicable. What then remains crucial is correcting the package declarations across the entire project in Eclipse – a task that might seem daunting at first.
The Issue
The issue arises when developers need a quick, effortless method to update all package declarations in the Package Explorer at once. Though Ctrl+Shift+O can be used to resolve imports by selecting the source, there’s a doubt whether the same shortcut can be applied to rectify package declarations.
In some cases, refactoring the packages may not be a feasible option as retaining their name and location becomes necessary. The adjustment required lies only in the package declaration of the Java source code.
Tackling the Issue
One of the strategies to handle this is leveraging the Problems view in Eclipse. When the package declarations seem out of sync, the Problems view displays all related invalid declarations. If this view is not readily visible, you can navigate to it via Window -> Show View -> Other… -> Problems (under the General tab).
The Problems view offers the provision to filter out specific issues, enabling developers to make the required corrections effortlessly. By picking the suitable Quick fix option from the context menu, the easily rectifiable problems can be sorted. This context menu is accessible by simply right-clicking.
However, keep in mind that knowing the correct quick fix option is key to resolving the issue, which could involve modifying either the class’s package declaration or its location. Regrettably, there’s no option to resolve the problem simultaneously over multiple units – quick fixes must be applied individually to each issue.
To focus exactly on this specific problem, configure the settings in the Problems view to exhibit errors containing the phrase “does not match the expected package” in their description. Screenshots supplementing the textual explanation assist in visually demonstrating this configuration.
In conclusion, while Eclipse doesn’t currently offer a single-step solution to update package declarations across an entire project, leveraging the Problems view and the Quick fix functionality can notably streamline the process, making it less daunting and more manageable.
Quick Solutions to Update Package Declarations in Eclipse
There are times during Java development in Eclipse when substantial amounts of source code have been imported, leading to an inapplicability of the existing package name. Making individual changes to each file seems like a daunting task. Here are a few straightforward solutions that can help update all package declarations in an entire project at once.
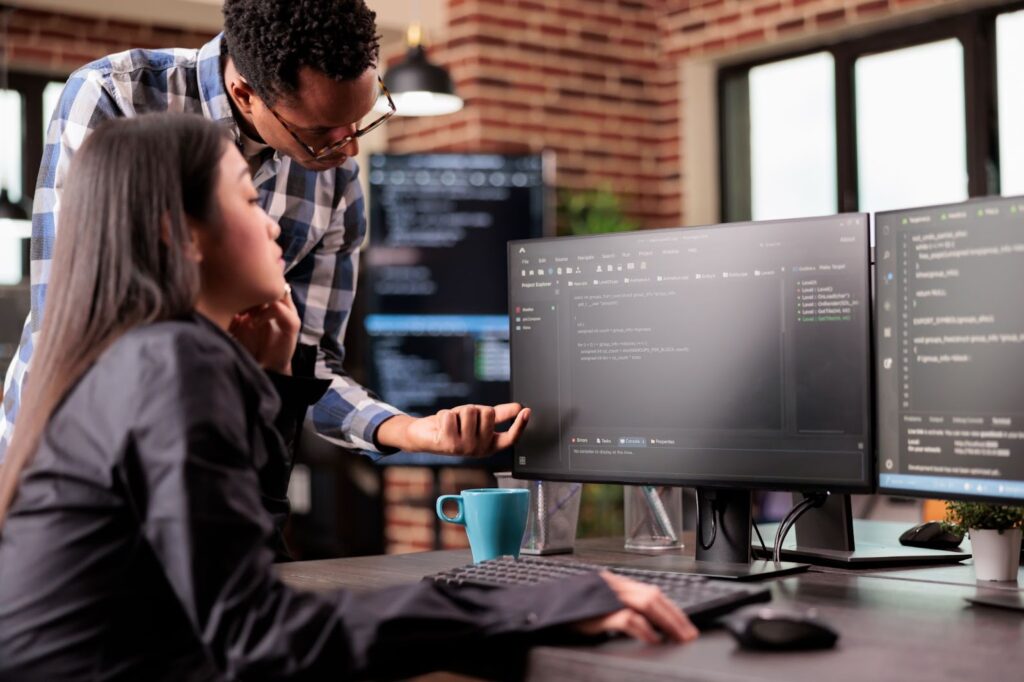
Strategy 1: Using Eclipse’s File Search and Replace
This approach is especially handy when artifacts, often generated automatically, are present alongside the source files.
Assume that “package abc;” has been used across 200 files, and this needs to be updated to “package com.aa.bb.cc.abc;”. The solution? Use Eclipse’s Search->File feature to locate “package abc;” in the required folder, package, or the entire workspace. Instead of merely searching, click on “Replace” and enter “package com.aa.bb.cc.abc;” as the replacement string. Apply the changes, and all instances of “package abc;” will be replaced with the new package name.
Strategy 2: Using Eclipse’s Refactor>Rename Feature
Another way to update the package name across all source files is to right-click on the package, and select Refactor > Rename. Enter the new package name in the dialog box that appears, and Eclipse will handle the rest.
Strategy 3: Creating a Bash Script
For those comfortable with bash scripting, creating a script to update the package names can be a powerful tool.
Here is a function that can be used to update the package names in all Java files within a directory:
function java-package-update {
for path in $(find $1 -type f -name "*.java"); do
D=$(dirname $path);
F=$(basename $path);
P=$(echo $D|tr '/' '.');
if egrep -q '^\s*package\s*' $path; then
sed -i '' '/^\s*package\s*/s/^\(\s*package\s*\)[^;]*\(;.*\)/\1 '$P'\2/' $path;
else
echo >&2 "no package in $path";
fi;
done;
}
Please note that the sed command on OSX requires the ‘’ parameter after -i. However, GNU sed does not need this parameter.
To use this script, simply copy it and execute it on the directory containing your source code. However, it’s always recommended to make a backup of your code before proceeding with scripts.
Strategy 4: Deleting the Default Package Declaration
Eclipse allows you to create a default package easily. Create a new class (New -> Class), erase the package field, and name the class as desired. Make sure the class is placed in the correct project. If a class is already in the wrong package, merely delete the package name from the top of the file and drag the file in the file viewer to the src folder itself.
In summary, there are multiple ways to update package declarations across an entire project in Eclipse. The right solution depends on specific use cases and familiarity with various tools and programming languages.
Creating a Default Package in Eclipse: Solutions and Best Practices
Understanding the Issue
As a novice Java developer, starting with a new project in Eclipse can seem a tad challenging. While initiating a project, it automatically generates the source folder (src) and JRE System Library by default. However, the creation of a default package is not always apparent.
When a developer proceeds to create a class under the ‘src’ directory without specifying a package, Eclipse might automatically name it after the project and prompt to include an additional package name. However, if the developer does not want to add specific package names to their classes, they might look for a way to create a default package in Eclipse.
Strategy to Create a Default Package
Creating a class and setting it to a default package is a simple process. Follow these steps:
- Navigate to ‘New’ -> ‘Class’;
- Erase the content in the package field;
- Assign a preferred name to the class;
- Ensure that the class is in the correct project.
For classes already residing in the wrong package:
- Delete the package name from the top of the file;
- Drag and drop the file to the src folder through the file viewer.
Despite this, it’s essential to consider the ramifications behind not specifying package names carefully.
Importance of Naming Packages
While this strategy can create a default package, it’s essential to understand why Eclipse prompts developers to add specific package names to their classes.
- Organization: Naming packages improves code organization. If all classes are dumped into a default package, it gets cluttered and nearly impossible to navigate through thousands of classes over time;
- Minimizing Naming Conflicts: If everything is stored in the default package, naming a new class would require checking if the name already exists to avoid naming conflicts;
- Easier Troubleshooting: Well-organized classes within named packages make it easier to troubleshoot and understand where a potential bug or issue might be coming from;
- Improved Readability: Categorized classes increase code readability and help other developers understand and navigate the codebase efficiently.
In conclusion, while creating a default package in Eclipse is a straightforward process, it’s good practice to name packages, primarily to keep the code organized, enhance readability, and minimize potential naming conflicts.
Conclusion
In conclusion, Eclipse’s Search->File feature provides a seamless solution for updating package declarations across multiple files within a project. By leveraging this tool, users can effortlessly transition from “package abc;” to “package com.aa.bb.cc.abc;” with precision and efficiency. This streamlined process underscores Eclipse’s commitment to enhancing developers’ productivity and workflow management within the IDE.