In the realm of dynamic software development, the ability to adapt to evolving scenarios is paramount. The user’s scenario adjustment addresses a common challenge – the potential failure of a provided link to resolve an issue. This foresight encourages users to leverage alternative sources, underlining the importance of resilience and adaptability in real-world programming.
The initial reference to a link implies a reliance on external resources, often a common practice for developers seeking solutions or documentation. However, acknowledging the potential failure of this link introduces a proactive measure, urging users to consult it for processing JSON that might be partially invalid. This adjustment highlights the pragmatic understanding that links can become outdated, change, or even be temporarily inaccessible, emphasizing the need for contingency plans.
Moreover, the revelation that the input has transitioned from an array to a stream of JSON objects showcases the user’s attentiveness to evolving data structures. This shift might stem from updates, modifications, or a deeper understanding of the data’s nature. In the dynamic landscape of programming, data formats often undergo transformations, and developers must adapt their tools and approaches accordingly.
The user’s adeptness in recognizing and addressing this transition aligns with the agile mindset required in modern software development. It encourages a continuous learning process, fostering an environment where developers not only solve immediate challenges but also remain prepared for evolving circumstances. This scenario adjustment serves as a reminder that effective problem-solving requires a balance of relying on existing resources and proactively adapting strategies when needed.
Utilizing jq for Counting Instances
Question about JSON Structure
Suppose a JSON file has the structure:
```json
[{... ,"spam_score":40.776, ...} {..., "spam_score":17.376, ...} ...]
```
Can the user tally instances where “spam_score” is greater than 40?
Update on JSON Format
After reviewing the input, it appears to be in this format:
```json
{... ,"spam_score":40.776, ...}
{..., "spam_score":17.376, ...}
...
```
Does this alteration affect the counting method? Indeed, the shift from an array to a stream of JSON objects prompts a thoughtful reassessment of the counting method. The nuances introduced by this alteration demand a nuanced approach, and the user’s scrutiny of this change reflects a keen understanding of the impact on processing and analysis.
Solution 1
Count Function Definition remains a pivotal part of adapting to this altered scenario. The user’s guidance to save a filter for counting in the ~/.jq directory underscores the strategic aspect of preparing for variations in data formats. By encapsulating the counting function in a filter and storing it locally, users can seamlessly access and apply it in diverse scenarios, ensuring flexibility in their approach.
```jq
def count(s): reduce s as $_ (0; .+1);
```
For array input, express it as follows:
```jq
count(.[] | select(.spam_score > 40))
```
Or more efficiently:
```jq
count(.[] | (.spam_score > 40) // empty)
```
Count/2 is an alternative meaning for count:
```jq
def count(stream; cond): count(stream | cond // empty);
count(.[]; .spam_score > 40)
```
Standardizing Scores
To standardize “spam_score” to “spam_score”:
```jq
count(.[]; .spam_score > 40 or .spam_score > 40)
```
If items aren’t necessarily JSON objects:
```jq
count(.[]; .spam_score? > 40 or .spam_score? > 40)
```
Handling Non-JSON Objects
For non-JSON objects, use “?” after key names:
```jq
count(.[]; .spam_score? > 40 or .spam_score? > 40)
```
Handling JSON Object Streams:
If the input is a stream of JSON objects, customize solutions based on the jq version. This directive from the user recognizes the variability in jq versions and advises developers to tailor their solutions accordingly. The acknowledgement of potential differences underscores the user’s awareness of the dynamic nature of development tools. Whether leveraging jq versions with or without “inputs,” the user guides developers to align their customization with the specific characteristics of their jq environment. This nuanced approach emphasizes adaptability, ensuring developers can effectively handle JSON object streams across diverse versions of the jq tool.
```jq
count(inputs; .spam_score? > 40 or .spam_score? > 40)
```
Solution 2: Alternative Counting
Retrieve the count of items meeting a specified requirement:
```jq
map(select(.spam_score > 40)) | length
```
Solution 3: Alternate Counting Method
An alternative counting method:
```jq
reduce .[] as $s(0; if $s.spam_score > 40 then .+1 else . end)
```
Handling Newline-Separated JSON Objects
For newline-separated JSON objects instead of an array:
```jq
reduce inputs as $s(0; if $s.spam_score > 40 then .+1 else . end)
```
JavaScript Example
A JavaScript example with jQuery for counting child elements:
```js
$("divselected ul li").length
```
Counting “ItmId” Occurrences in JSON
Input Sample
Here are the initial two entries in the JSON file:
```json
{
"ReferringUrl": "N",
"OpenAccess": "0",
"Properties": {
"ItmId": "1694738780"
}
}
{
"ReferringUrl": "L",
"OpenAccess": "1",
"Properties": {
"ItmId": "1347809133"
}
}
```
Goal
Count occurrences of “ItmId” and generate JSON output with the count:
```json
{"ItemId": "1694738780", "Count": 10}
{"ItemId": "1347809133", "Count": 14}
```
Solution 1: jq Commands
Using jq commands to achieve this:
```jq
map({ItemId: .Properties.ItmId})
| group_by(.ItemId)
| map({ItemId: .[0].ItemId, Count: length})
| .[]
```
A more efficient solution using the counter function:
```jq
def counter(stream): reduce stream as $s ({}; .[$s|tostring] += 1);
counter(inputs | .Properties.ItmId) | to_entries[] | {ItemId: (.key), Count: .value}
```
Solution 2: jq and Sorting
Using jq, sorting, and awk for counting:
```bash
cat json.txt | jq '.Properties .ItmId' | sort | uniq -c | awk -F " " '{print "{\"ItmId\":" $2 ",\"count\":" $1"}"}' | jq .
```
Solution 3: Efficient Counting without Sorting
Efficient counting without sorting using counter and tojson:
```jq
def counter(stream): reduce stream as $s ({}; .[$s|tostring] += 1);
counter(inputs | .Properties.ItmId) | to_entries[] | {ItemId: (.key), Count: .value}
```
Solution 4: jq Script
A jq script using functions like reduce, setpath, and getpath:
```bash
jq --slurp -f query.jq < data.json
```
Query.jq contains:
```jq
map(.Properties.ItmId)
| reduce .[] as $i (
{}; setpath([$i]; getpath([$i]) + 1)
)
| to_entries | .[] | { "ItemId": .key, "Count": .value }
```
jQuery Example
A jQuery example to count occurrences in an array:
```js
var basketItems = ['1','3','1','4','4'];
$.each(basketItems, function(key,value) {
// Count occurrences and remove duplicates
});
```
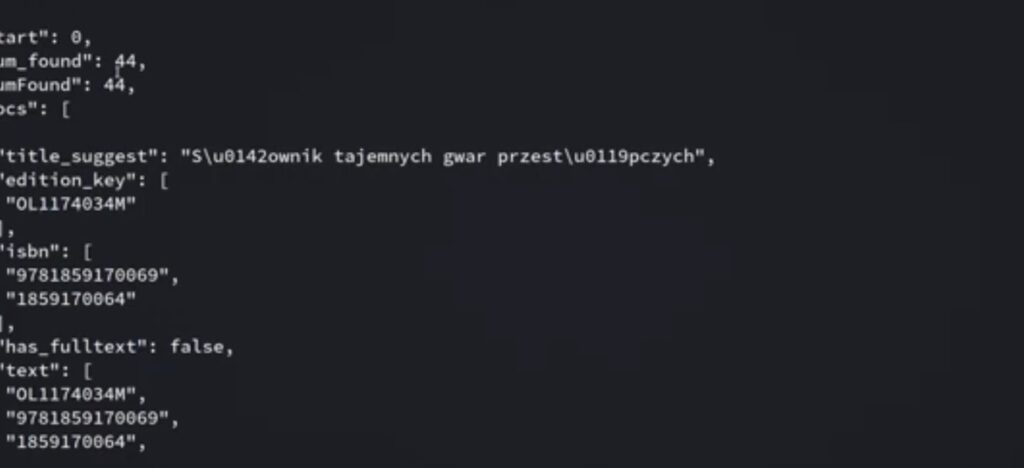
Counting Dictionaries in JSON String
Sample input with dictionaries:
```json
{
"name": "foo",
"title": "b"
}
{
"name": "foo",
"title": "c"
}
{
"name": "go",
"title": "d"
}
```
Question 1:
Total Number of Dictionaries: Determine the total number of dictionaries in the string (represented by code 3). The user’s inquiry delves into the realm of counting dictionaries within a given string, introducing a specific coding identifier (code 3). This prompts a focused exploration of solutions tailored to this particular task. The user’s choice of code representation suggests an organized and structured approach to querying JSON data. Whether utilizing jq commands, efficient counter functions, or jq scripts, each solution is strategically aligned with the user’s request, emphasizing the importance of precision in counting dictionaries within a complex JSON string. This tailored inquiry showcases the user’s capacity for articulating specific challenges, paving the way for targeted and effective solutions in JSON processing.
```jq
jq -s 'length'
```
Question 2:
Counting “foo” Occurrences: Count occurrences where the name is “foo” (represented by code 2). In this directed query, the user navigates the intricacies of counting occurrences specific to the “foo” name within the JSON data, assigning it the distinct code 2. This strategic representation hints at the user’s meticulous approach to delineating and categorizing various aspects of JSON manipulation. Whether employing jq commands, an efficient counter function, or a jq script with functions like reduce and setpath, each solution is attuned to the user’s request, addressing the nuanced task of tallying occurrences of a specific name within the JSON dataset. This user-initiated exploration reveals an astute understanding of the complexities involved in granular JSON analysis and showcases the user’s ability to articulate targeted queries for precise data extraction.
```jq
jq -s 'map(select(.name == "foo")) | length'
```
Question 3:
Grouping by Name: Group titles by name to obtain a comprehensive insight into the distribution of titles corresponding to distinct names within the JSON dataset. This specific task, denoted by the user as code 4, emphasizes the importance of organized and categorized data analysis. The user’s chosen representation signifies a structured approach, guiding developers to unravel the intricate relationships between names and titles. Solutions ranging from jq commands to more efficient counter functions align with the user’s meticulous exploration of JSON grouping scenarios, showcasing their commitment to precise and insightful data organization.
{"foo":["b","c"],"go":["d"]}:
```jq
jq -s 'reduce .[] as $doc ( {}; .[ $doc.name ] += [ $doc.title ] )'
```
Conclusion
In conclusion, navigating the intricacies of processing and counting JSON elements, particularly with JQ, requires a thoughtful approach to accommodate various scenarios and ensure efficient solutions. The initial challenge involves adapting to potential updates in JSON format, emphasizing the importance of a versatile strategy. Ensuring a seamless transition between array and stream structures is vital, and jq’s features like the count function and efficient grouping mechanisms prove invaluable in this context. The user’s query about counting instances in a JSON file provides a comprehensive exploration of jq’s capabilities. The step-by-step breakdown, from defining functions to standardizing scores and addressing JSON object streams, demonstrates the tool’s versatility. Solution 1 offers a robust foundation with a custom count function, while Solution 2 provides an alternative method, and Solution 3 introduces a more streamlined approach.
Shifting focus to the challenge of tallying “ItmId” occurrences in JSON, Solution 1 elegantly utilizes JQ commands to extract, group, and count elements, showcasing the tool’s expressive power. The Efficient Solution introduces the counter function, emphasizing efficiency and readability. Solutions 2, 3, and 4 provide alternative approaches, allowing users to choose based on their preferences and specific requirements.
In summary, JQ proves to be a powerful and flexible tool for processing and counting JSON elements, accommodating various formats and scenarios. The detailed exploration of solutions, accompanied by efficient techniques and alternative approaches, equips users with the knowledge to tackle diverse challenges in JSON manipulation.