Docker has become an essential tool for developers, allowing them to easily build, ship, and run applications in a self-contained environment. With the help of Dockerfiles, developers can automate the process of building and deploying applications, saving valuable time and effort.
One of the most common tasks in a Dockerfile is copying files from the host machine into the container. While it may seem like a simple task, copying files from parent directories in a Dockerfile can be tricky and confusing for many developers. In this article, we will delve deep into the different techniques and best practices for copying files from parent directories in a Dockerfile.
What is Dockerfile COPY Instruction?
Before we dive into the specifics of copying files from parent directories, let’s first understand the COPY instruction in a Dockerfile. The COPY instruction is used to copy files or directories from the host machine into the container at build time. It takes two arguments – the source (host machine) and the destination (container).
The basic syntax for COPY instruction is as follows:
COPY
For example, if we want to copy a file named app.js from the host machine into the /app directory in the container, the COPY instruction would look like this:
COPY app.js /app/
It is important to note that the path should always be relative to the image’s working directory. If the directory does not exist, it will be created automatically. If the file exists, it will be overwritten with the newly copied file.
Now, let’s move on to the main topic of this article – copying files from parent directories.
Techniques for Copying Files from Parent Directories
Using Absolute Paths
One of the most common methods for copying files from parent directories is by using absolute paths. This technique involves specifying the full path of the file or directory in both the source and destination arguments of the COPYinstruction.
Let’s consider an example where we have a project structure like this:
project
├── Dockerfile
└── src
├── app.js
└── package.json
If we want to copy the package.json file from the src directory into the container, our COPY instruction would look like this:
COPY /project/src/package.json /app/
Here, we are specifying the full path of the file in the source argument and the relative destination in the destination argument. It is worth noting that the leading slash / in the source path indicates the root directory.
This method works well when you have a simple project structure with a few files and directories. However, as your project grows, managing these long and complex paths can become cumbersome and error-prone.
Using Build Context
Another way to copy files from parent directories is by using the build context. The build context is the set of files located in the same directory as the Dockerfile and its subdirectories. When building an image, the build context is sent to the Docker daemon, allowing it to access any files or directories within it.
To use the build context for copying files from parent directories, we need to make sure that the files we want to copy are present in the build context. Going back to our previous example, if we want to copy the package.json file from the srcdirectory, we need to make sure that the src directory is included in the build context.
We can do this by specifying the build context in the docker build command using the -f flag. In our case, the command would look like this:
docker build -t app-image -f Dockerfile .
The . at the end of the command indicates that the current directory is the build context. Once the build context is set, we can use relative paths in the COPY instruction to copy files or directories from parent directories.
In our example, the COPY instruction would look like this:
COPY src/package.json /app/
This method is more convenient and less error-prone compared to using absolute paths. However, it still requires us to manage the build context and make sure that the necessary files and directories are present in it.
Using Build Arguments
Docker allows us to pass build arguments to the docker build command using the –build-arg flag. These arguments can be used inside the Dockerfile during the build process. We can leverage this feature to copy files from parent directories without worrying about the build context or managing long paths.
To use build arguments for copying files, we need to declare them in the Dockerfile using the ARG instruction. The syntax for ARG instruction is as follows:
ARG [=]
For example, if we want to declare a build argument named APP_PATH, we can do it like this:
ARG APP_PATH
Now, we can use this argument in our COPY instruction as follows:
COPY $APP_PATH/package.json /app/
Notice that we are using the $ symbol to reference the build argument in the COPY instruction. This method allows us to keep our Dockerfile clean and manageable by avoiding long and complex paths.
We can pass the value for this build argument using the –build-arg flag in the docker build command. In our case, the command would look like this:
docker build -t app-image --build-arg APP_PATH=src .
Here, we are setting the value for the APP_PATH argument as src, which is the parent directory containing our package.json file.
Using this technique, we can easily copy files from different parent directories by changing the build argument value. This method is especially useful in cases where the parent directory name changes frequently or when dealing with complex project structures.
Best Practices for Copying Files from Parent Directories
Now that we have covered the different techniques for copying files from parent directories, let’s discuss some best practices that you should keep in mind while using these techniques.
Use Relative Paths
No matter which technique you use for copying files from parent directories, it is always recommended to use relative paths in the COPY instruction. Using relative paths makes your Dockerfile more portable and independent of the host machine’s directory structure.
Additionally, if you decide to change your project structure in the future, you won’t have to make any modifications to the Dockerfile as long as the relative paths remain the same.
Keep Your Directory Structure Organized
Having a well-organized directory structure is crucial for effective Dockerfile management. Make sure to keep all the necessary files and directories within the build context so that they can be easily accessed by the Docker daemon.
Moreover, avoid creating long and complex directory structures as they can make it difficult to manage the paths in your COPY instructions. Instead, follow a simple and intuitive grouping of files and directories, making it easier to navigate and copy them into the container.
Leverage Build Arguments for Changing Parent Directories
As mentioned earlier, using build arguments can be a powerful tool for copying files from parent directories. By setting the value for the build argument at build time, you can easily change the parent directory without making any modifications to the Dockerfile.
This method is particularly useful when dealing with complex project structures where the parent directory name may change frequently. Instead of manually changing the paths in your COPY instructions, you can simply pass a different value for the build argument during the build process.
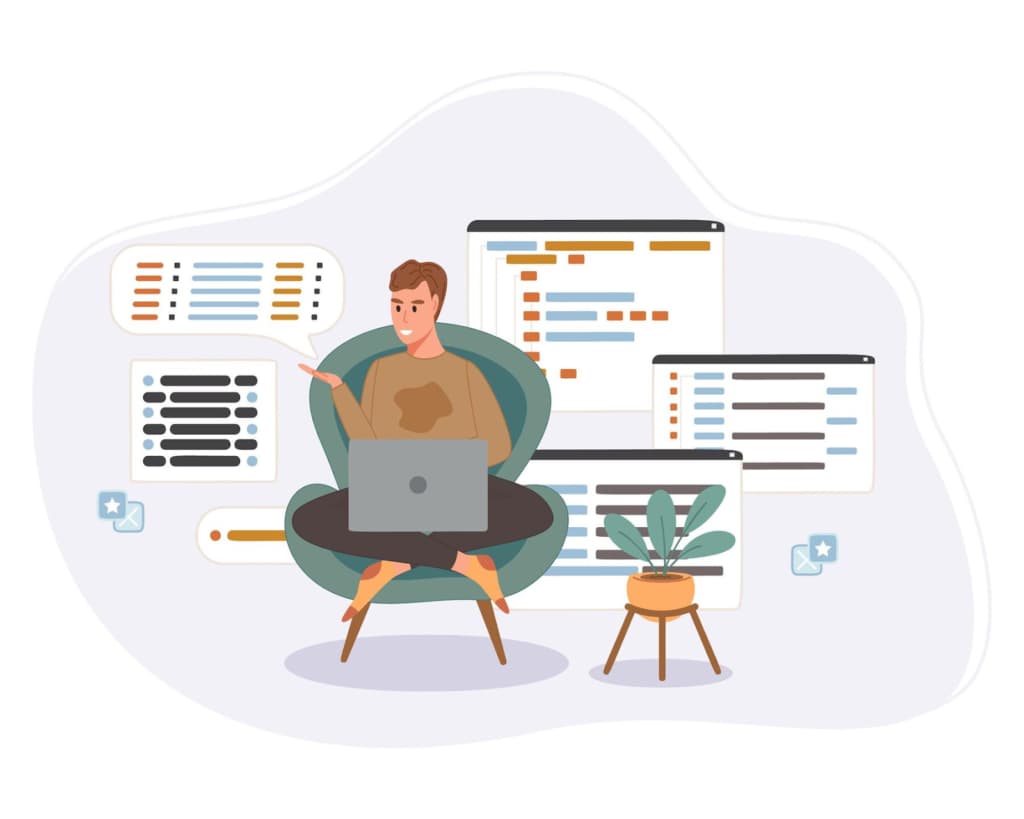
Examples and Use Cases
To further solidify our understanding of copying files from parent directories, let’s take a look at some examples and use cases.
Copying Multiple Files
In some cases, you may need to copy multiple files from different parent directories into the container. For example, if your application requires multiple configuration files, you can use the same techniques discussed earlier to copy them into the container.
Let’s consider a scenario where we have the following project structure:
project
├── Dockerfile
└── config
├── app_config.json
└── db_config.json
To copy both the app_config.json and db_config.json files from the config directory into the /app/config directory in the container, we can use the COPY instruction like this:
COPY config/app_config.json /app/config/
COPY config/db_config.json /app/config/
Here, we are using relative paths to specify the source and destination for each file.
Alternatively, we can also use build arguments to make the COPY instruction more concise and manageable. We can declare the build argument in our Dockerfile like this:
ARG CONFIG_PATH
And then use it in our COPY instruction as follows:
COPY $CONFIG_PATH/app_config.json /app/config/
COPY $CONFIG_PATH/db_config.json /app/config/
Now, we can pass different values for the CONFIG_PATH argument to copy files from different parent directories.
Dealing with Nested Directories
In some cases, your project structure may contain nested directories, making it difficult to navigate and copy files from them. In such scenarios, you can use wildcards in the COPY instruction to copy files from multiple levels of parent directories.
For example, if we have a project structure like this:
project
├── Dockerfile
└── src
├── app.js
└── modules
├── module1
│ └── index.js
└── module2
└── index.js
And we want to copy all the index.js files present in the src/modules directory into the container, we can use the following COPY instruction:
COPY src/modules/**/*.js /app/
Here, the wildcard indicates that any number of nested directories can exist between modules and js. This will copy all the index.js files from both module1 and module2 directories into the /app directory in the container.
Using Environment Variables
Another way to copy files from parent directories is by using environment variables in the Dockerfile. Environment variables can be used to store values that are accessible during the build process and at runtime. You can leverage this feature to set the source and destination paths for the COPY instruction dynamically.
Let’s consider a scenario where we want to copy a file named config.json located in the config directory of our project into the /app/config directory in the container. We can declare an environment variable CONFIG_PATH in our Dockerfile and use it in the COPY instruction as follows:
ENV CONFIG_PATH=config
COPY $CONFIG_PATH/config.json /app/config/
Now, during the build process, we can set the value for the CONFIG_PATH environment variable using the –build-arg flag. For example, our docker build command would look like this:
docker build -t app-image --build-arg CONFIG_PATH=config .
Here, we are setting the value for the CONFIG_PATH variable as config, which is the parent directory of our config.json file.
Conclusion
In this article, we have explored different techniques and best practices for copying files from parent directories in a Dockerfile. We started by understanding the basics of the COPY instruction and then moved on to more advanced techniques such as using build arguments and environment variables.