In TypeScript, effectively managing loop execution is essential for optimizing code performance. To avoid the automatic instantiation issues that can arise with forEach loops, it’s practical to declare any necessary variables before the loop starts. By opting for a traditional for loop instead of forEach, developers gain direct control over the loop’s execution, including the ability to stop it whenever specific conditions are met. This method simplifies the process of controlling loop behavior, making it a straightforward choice for those seeking to improve their code’s efficiency and responsiveness.
Strategies for Breaking ForEach Loops in TypeScript
The struggle lies in terminating the loop under specific circumstances in the code snippet provided below.
isVoteTally(): boolean {
let count = false;
this.tab.committee.ratings.forEach(element => {
const _fo = this.isEmptyOrNull(element.ratings.finalOutcome.finaloutlook);
const _foreign = this.isEmptyOrNull(element.ratings.finalOutcome.foreign);
const _local = this.isEmptyOrNull(element.ratings.finalOutcome.local);
const _tally = element.ratings.finalOutcome.voteTally.maj + element.ratings.finalOutcome.voteTally.dis;
if (_fo == false && _foreign == false && _local == false) {
if (_tally > 0) {
**return count = false;**
}
} else {
if (_tally < 0) {
**return count = false;**
}
}
});
return count;
}
Currently encountering challenges in breaking the code and extracting the boolean value from the area marked with a star. Assistance from anyone familiar with this task would be appreciated.
Solution 1:
The construct “this.tab.committee.ratings.forEach” is not an operator.
TypeScript code is designed for readability.
For a more structured approach, implement a loop with “for” styling like this:
for (let a of this.tab.committee.ratings) {
if (something_wrong) break;
}
It’s advisable to avoid using jQuery-style coding in Angular as it’s not efficient.
Solution 2:
It’s not feasible to break out of a forEach() loop conventionally.
However, if you need to exit the loop while returning false, you can utilize array.every(). Alternatively, to obtain a true return, you can employ Array.some().
this.tab.committee.ratings.every(element => {
const _fo = this.isEmptyOrNull(element.ratings.finalOutcome.finaloutlook);
const _foreign = this.isEmptyOrNull(element.ratings.finalOutcome.foreign);
const _local = this.isEmptyOrNull(element.ratings.finalOutcome.local);
const _tally = element.ratings.finalOutcome.voteTally.maj + element.ratings.finalOutcome.voteTally.dis;
if (_fo == false && _foreign == false && _local == false) {
if (_tally > 0) {
**return count = false;**
}
} else {
if (_tally < 0) {
**return count = false;**
}
}
});
Solution 3:
Executing the break statement outside of a loop won’t trigger any action, thus failing to terminate it. To address this issue, consider using a standard for loop instead. Rest assured, choosing this approach won’t invite any ridicule.
Solution 4:
A more efficient strategy is to employ a “for” loop. This method allows for breaking the loop and promptly returning a value upon discovery, leading to a notably streamlined solution.
for (element of this.tab.committee.ratings) {
// and here you use your element, when you return a values it stops the cycle
if (element === something){
return element;
}
}
Breaking a forEach Loop in TypeScript is not straightforward. However, you can achieve it by utilizing Array.every() if you intend to return false while breaking the loop. Conversely, if you aim to return true, Array.some() can be utilized.
Here’s a code sample:
const _fo = this.isEmptyOrNull(element.ratings.finalOutcome.finaloutlook);
const _foreign = this.isEmptyOrNull(element.ratings.finalOutcome.foreign);
const _local = this.isEmptyOrNull(element.ratings.finalOutcome.local);
const _tally = element.ratings.finalOutcome.voteTally.maj + element.ratings.finalOutcome.voteTally.dis;
if (_fo === false && _foreign === false && _local === false) {
// Your feedback or further code goes here
}
In this code, you can adapt the conditions and the subsequent actions based on your specific requirements.
Nested ForEach Loop Control in TypeScript
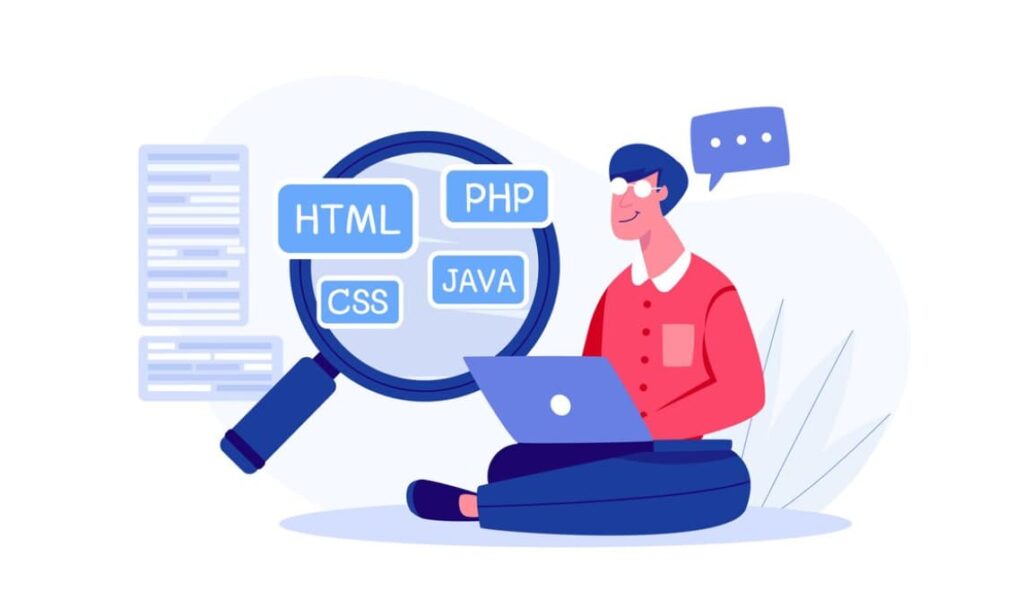
Facing an issue in TypeScript while trying to utilize a break statement within nested forEach loops. Error message “jump target cannot cross function boundary” appears. Seeking a solution to halt the forEach loop upon meeting a specific condition.
groups =[object-A,object-B,object-C]
groups.forEach(function (group) {
// names also an array
group.names.forEach(function (name) {
if (name == 'SAM'){
break; //can not use break here it says jump target cannot cross function boundary
}
}
}
Solution 1:
The forEach function iterates through each element in the array, executing the provided function for each, without an option to prematurely terminate the loop. Nevertheless, you can utilize the return statement to exit the function after a single iteration if required.
To break out of the loop, consider using the for..of loop construct as an alternative approach.
Example:
for(let name of group.names){
if (name == 'SAM') {
break;
}
}
Solution 2:
In order to halt a loop, the return statement should be utilized since the forEach method doesn’t support breaking out of the loop.
Example:
groups =[object-A,object-B,object-C]
groups.forEach(function (group) {
// names also an array
group.names.forEach(function (name) {
if (name == 'SAM'){
return; //
}
}
}
Solution 3:
To iterate over the keys of an object and perform operations, you can utilize Object.keys(fields).forEach(). This allows you to check for empty or null values and handle them accordingly.
Object.keys(fields).forEach(function (key, index) {
if (fields[key] !== null && fields[key].toString().trim().length === 0) {
console.log('error');
return;
}
});
Solution 4:
Despite the inability to comment due to insufficient reputation, a compile error was encountered when attempting Mahmoodvcs’ solution, which indicated that “name” could not be found.
To prevent unintended variable instantiation in a forEach loop, it is advisable to declare the variable before entering the loop. While this approach may seem straightforward, it’s essential to stay cautious about potential issues. Hopefully, this tip proves helpful to others.
Consider the following code snippet, which closely resembles forEach but guarantees the desired behavior:
for(let name of group.names){
if (name == 'SAM') {
break;
}
}
The scenario presented included some variation. It involved including a return statement within a forEach loop block, with the intention for the return to affect the enclosing function rather than the forEach loop itself. Fortunately, no error was thrown, and reading this post beforehand was considered fortunate. Without this prior knowledge, there could have been an entire day spent in frustration and confusion. Initially, the code was structured as follows:
Object.keys(this.ddlForms).forEach(x => {
if (!(!this.ddlForms[x].filterControl.value || this.ddlForms[x].filterControl.value[0] == 'All' || this.ddlForms[x].filterControl.value.some(y => y == data[this.ddlForms[x].fieldName]))) {//does not meet any of these three conditions
return false;
}
});
to:
for(let x of Object.keys(this.ddlForms)) {
if (!(!this.ddlForms[x].filterControl.value || this.ddlForms[x].filterControl.value[0] == 'All' || this.ddlForms[x].filterControl.value.some(y => y == data[this.ddlForms[x].fieldName]))) {//does not meet any of these three conditions
return false;
}
}
How to manage control flow across nested forEach loops: ForEach loops don’t inherently support break or return statements for exiting early. While some documentation may suggest that return works within forEach, it typically doesn’t affect the loop’s behavior. Instead, consider using the .some method which guarantees a return once the first item is found. This is particularly useful if you need to exit the loop prematurely based on certain conditions. By leveraging .some, you can efficiently handle control flow within nested forEach loops and ensure the desired behavior.
Strategies for Interrupting Execution Flows in TypeScript
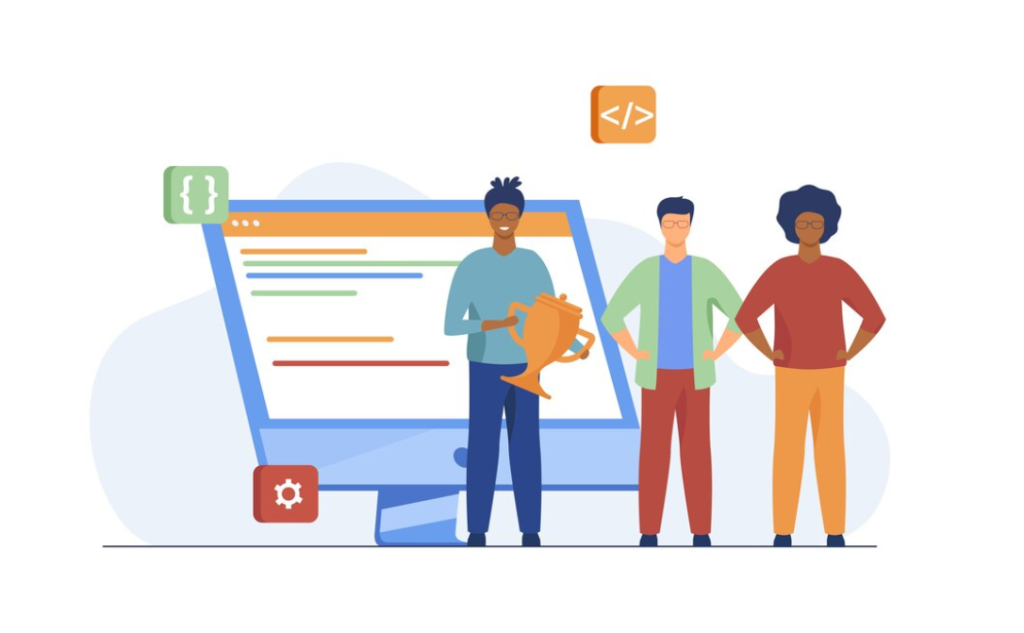
Challenges are being faced when attempting to stop execution flow based on specific conditions in TypeScript. The requirement is to display an error message that should be dismissible by users, allowing them to remove the error notification without encountering any issues.
Furthermore, when the “ok” button is clicked, the error message should not be removable by user interaction but should instead disappear on its own after a predetermined period. What approaches can be taken to implement this behavior within TypeScript code?
public findVerticesBelow(vertexId: number) {
// debugger
this.recussionTime++;
if(this.recussionTime <= 150){
console.log(this.recussionTime)
if (!this.vertexIdList.includes(vertexId)) {
this.vertexIdList.push(vertexId)
}
let tempvertexIdList: Array = new Array();
this.edges.forEach(edge => {
if (edge.fromId == vertexId) {
tempvertexIdList.push(edge.toId);
}
})
tempvertexIdList.forEach(tempvertexId => {
this.findVerticesBelow(tempvertexId)
});
}else{
// debugger
console.log("AAAAAAAAAAAAAAA")
// alert("You are having a recursive loop please remove it before trying to remove nodes")
// alert("ASCDFVGBHN")
}
}
Currently, obtaining the desired boolean value by breaking the code remains a challenge. Assistance from someone knowledgeable in this matter would be greatly appreciated.
Solution 1:
Utilizing a method that may not be conventionally correct can still be an option.
let a=[1,2,3,3,4,4,4];
try{
a.forEach((x,y)=>{
console.log(x);
if(x==4){
throw "break";
}
})
}catch(e){
console.log(e);
}
Solution 2:
Terminating a forEach loop within JavaScript or TypeScript files directly is not achievable. To effectively terminate a loop using the “break” keyword, opting for a for loop is advisable. For comprehensive details, visiting the suggested link would be beneficial.
Solution 3:
To circumvent the limitations of the forEach loop, such as the inability to break normally, employing a for loop is a viable strategy.
Several characteristics of the forEach loop might not be commonly understood:
- The “return” statement does not halt the looping process;
- It is not possible to use ‘break’;
- Utilization of ‘continue’ is also not feasible.
For a deeper understanding of the forEach loop in JavaScript, engaging with resources such as the article available on Medium’s Front-End Weekly could provide valuable insights.
Alternatively, for scenarios requiring loop termination, leveraging Array.every() in conjunction with returning false is a method.
For cases necessitating a return value of true, the use of Array.some() is recommended.
In the context of Angular and TypeScript, breaking out of a forEach loop is not straightforward. Instead, a for loop should be employed. There are three lesser-known facts regarding the forEach loop that are crucial to acknowledge: the ineffectiveness of “return” in stopping loops, and the inability to use ‘break’ or ‘continue’.
Conclusion
In conclusion, understanding how to effectively manipulate loops in TypeScript is an invaluable skill for any programmer. This guide provides a comprehensive look into managing the ‘jump target cannot cross function boundary’ error, providing advanced tips and insights on how to handle it. Whether it’s breaking/continuing across nested forEach loops or compiling and executing a TypeScript program in Linux, mastering these concepts can largely streamline your programming journey in TypeScript.